如何在python中调用AES
时间: 2023-08-29 07:04:38 浏览: 46
要在 Python 中使用 AES 加密或解密数据,可以使用 `pycryptodome` 模块。`pycryptodome` 是 PyCrypto 的一个分支,提供了与 Python 3.x 兼容的版本。
首先,需要使用以下命令安装 `pycryptodome` 模块:
```
pip install pycryptodome
```
然后,您可以使用以下代码示例进行 AES 加密和解密:
```python
from Crypto.Cipher import AES
import base64
# 将密钥和待加密的数据补齐为 AES 块大小的倍数
def pad(s):
return s + b"\0" * (AES.block_size - len(s) % AES.block_size)
# 加密函数
def encrypt(message, key):
message = pad(message)
iv = b"1234567812345678" # 初始化向量必须为 16 个字节
cipher = AES.new(key, AES.MODE_CBC, iv)
ciphertext = cipher.encrypt(message)
return base64.b64encode(ciphertext)
# 解密函数
def decrypt(ciphertext, key):
iv = b"1234567812345678" # 初始化向量必须为 16 个字节
cipher = AES.new(key, AES.MODE_CBC, iv)
message = cipher.decrypt(base64.b64decode(ciphertext))
return message.rstrip(b"\0")
# 测试代码
key = b"1234567890123456" # 密钥必须为 16、24 或 32 个字节
message = b"Hello, World!"
encrypted_message = encrypt(message, key)
decrypted_message = decrypt(encrypted_message, key)
print("明文:", message)
print("加密后:", encrypted_message)
print("解密后:", decrypted_message)
```
其中,`encrypt` 函数使用 AES 密码模式(CBC)对数据进行加密,`decrypt` 函数使用同样的方式进行解密。需要注意的是,密钥长度必须为 16、24 或 32 个字节,且初始化向量必须为 16 个字节。本示例中使用的是 CBC 模式,您也可以根据需要使用其他模式(如 ECB、CFB 或 OFB)。
相关推荐
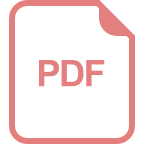














