Python如何定义字符串指针
时间: 2023-11-19 10:07:48 浏览: 253
在Python中,字符串是不可变的,因此没有字符串指针的概念。在Python中,可以直接使用字符串变量来引用和操作字符串。例如:
```
str1 = "hello"
print(str1[0]) # 输出结果为:h
```
如果需要在函数中传递字符串的引用,可以直接传递字符串变量。例如:
```
def func(str):
print(str)
str1 = "hello"
func(str1) # 输出结果为:hello
```
相关问题
python 字符串 转字符指针
在 Python 中,字符串是不可变的。因此,直接将字符串转换为字符指针是不可行的。然而,如果你需要将字符串传递给 C 语言函数或库,则可以使用 `ctypes` 模块来实现。下面是一个示例:
```python
import ctypes
# 将字符串转换为字节串
string = "Hello, World!"
bytes_string = string.encode('utf-8')
# 创建一个字符指针
char_pointer = ctypes.c_char_p(bytes_string)
# 在 C 语言函数中使用字符指针
my_c_function(char_pointer)
```
请确保你已经定义了 `my_c_function`,它是一个接受 `char*` 类型参数的 C 语言函数。这样,你就可以将 Python 字符串传递给 C 语言函数,并在 C 代码中使用字符指针了。
python给定字符串str1和str2,求str1的字串中含有str2所有字符的最小字符串长度。
可以使用滑动窗口的思想来解决该问题。具体步骤如下:
1. 定义两个指针left和right,分别表示滑动窗口的左右端点,初始值均为0。
2. 定义一个字典need,用于记录str2中每个字符出现的次数。
3. 定义一个计数器count,用于记录滑动窗口中已经包含了str2中多少个不同的字符。
4. 定义一个变量min_len,用于记录最小的包含str2所有字符的子串长度,初始值为正无穷大。
5. 开始循环,当右指针小于str1的长度时:
a. 如果str1[right]在need中出现过,将need[str1[right]]的值减一,并判断need[str1[right]]是否等于0,如果是,则将count加一。
b. 将右指针右移一位。
c. 当count等于need的长度时,说明滑动窗口已经包含了str2的所有字符,计算当前子串的长度,并将min_len更新为当前子串长度和min_len中的较小值。
d. 如果str1[left]在need中出现过,将need[str1[left]]的值加一,并判断need[str1[left]]是否大于0,如果是,则将count减一。
e. 将左指针右移一位。
6. 返回min_len即可。
代码实现如下:
```
def min_substring(str1, str2):
need = {}
for ch in str2:
need[ch] = need.get(ch, 0) + 1
left = right = 0
count = 0
min_len = float('inf')
while right < len(str1):
if str1[right] in need:
need[str1[right]] -= 1
if need[str1[right]] == 0:
count += 1
right += 1
while count == len(need):
if right - left < min_len:
min_len = right - left
if str1[left] in need:
need[str1[left]] += 1
if need[str1[left]] > 0:
count -= 1
left += 1
return min_len
```
测试代码:
```
print(min_substring('abcd', 'bc')) # 2
print(min_substring('adbecf', 'abc')) # 3
print(min_substring('a', 'a')) # 1
print(min_substring('aaaaa', 'a')) # 1
print(min_substring('ab', 'a')) # 1
```
阅读全文
相关推荐
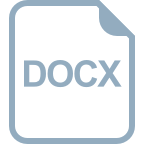
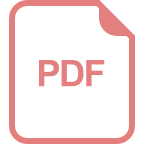
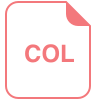




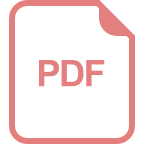
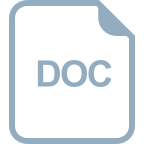
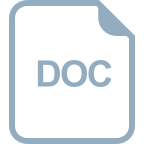
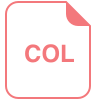
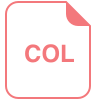



