如何在字符串数组中删除元素
时间: 2024-01-27 14:07:07 浏览: 212
在许多编程语言中,可以使用以下方法删除字符串数组中的元素:
1. 使用删除函数/方法。许多编程语言都有内置的删除函数/方法来删除数组中的元素。例如,在Python中,可以使用`del`或`.pop()`方法删除元素。在JavaScript中,可以使用`.splice()`方法删除元素。
2. 创建一个新数组。可以创建一个新数组,将要保留的元素复制到新数组中,以便从原始数组中删除元素。例如,在Java中,可以创建一个新数组并使用`System.arraycopy()`方法将要保留的元素复制到新数组中。在PHP中,可以使用`array_filter()`函数来过滤出要保留的元素并创建新数组。
以下是在Python中使用`.pop()`方法删除字符串数组中的元素的示例代码:
```
my_list = ["apple", "banana", "cherry", "orange"]
my_list.pop(1) # Removes "banana"
print(my_list) # Output: ["apple", "cherry", "orange"]
```
注意:在删除元素之后,数组的长度会减少。因此,在使用循环迭代数组时,要注意索引的变化。
相关问题
在c语言中如何在字符串数组中删除元素
在 C 语言中,字符串数组是一个字符指针数组,因此删除元素需要涉及指针操作。具体的实现方法可以通过以下步骤来完成:
1. 找到要删除的字符串在字符串数组中的位置。
2. 将该位置后面的所有字符串向前移动一个位置。
3. 将最后一个字符串置为空字符串。
以下是一个简单的示例代码,可以实现在字符串数组中删除指定位置的字符串:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 10
int main() {
char str_array[MAX_SIZE][100] = {"hello", "world", "this", "is", "a", "test"};
int pos = 3;
// 找到要删除的字符串在数组中的位置
int len = sizeof(str_array) / sizeof(str_array[0]);
if (pos >= len || pos < 0) {
printf("Invalid position!\n");
return 0;
}
// 将该位置后面的所有字符串向前移动一个位置
for (int i = pos; i < len - 1; i++) {
strcpy(str_array[i], str_array[i + 1]);
}
// 将最后一个字符串置为空字符串
memset(str_array[len - 1], '\0', sizeof(str_array[len - 1]));
// 输出删除后的字符串数组
for (int i = 0; i < len - 1; i++) {
printf("%s ", str_array[i]);
}
return 0;
}
```
在上面的示例代码中,我们首先定义了一个字符串数组 `str_array`,并且指定了数组的最大长度为 10。然后我们指定了要删除的字符串在数组中的位置 `pos`,这里我们选择删除第 4 个字符串 "is"。接着我们通过遍历数组将该位置后面的所有字符串向前移动一个位置,并将最后一个字符串置为空字符串。最后输出删除后的字符串数组。
java字符串数组删除指定元素
### 回答1:
您好,针对您的问题,可以使用Java中的ArrayList来进行元素删除操作。可以先将字符串数组转换为ArrayList,然后使用ArrayList的remove方法来删除指定元素。具体代码示例如下:
```
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
// 初始化字符串数组
String[] strArray = {"a", "b", "c", "d", "e", "f"};
// 将字符串数组转换为ArrayList
ArrayList<String> arrayList = new ArrayList<>(Arrays.asList(strArray));
// 删除指定元素
String target = "c";
arrayList.remove(target);
// 输出删除后的结果
System.out.println(arrayList);
}
}
```
运行以上代码,输出结果为:[a, b, d, e, f],可以看到,指定元素"c"已经被成功删除了。
### 回答2:
要删除Java字符串数组中的指定元素,可以按照以下步骤进行操作:
1. 创建一个新的字符串数组,长度比原来的数组少1。
2. 使用`for`循环遍历原数组,逐个比较数组元素是否与指定元素相同。
3. 如果元素相同,则跳过不进行复制;如果不同,则将元素复制到新数组中。
4. 完成循环后,新数组中就是删除指定元素后的结果。
下面是一个示例代码:
```java
public class Main {
public static void main(String[] args) {
String[] arr = {"apple", "banana", "orange", "lemon"};
String target = "orange";
String[] newArr = new String[arr.length - 1];
int newIndex = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i].equals(target)) {
continue;
}
newArr[newIndex] = arr[i];
newIndex++;
}
// 打印删除元素后的新数组
for (String str : newArr) {
System.out.println(str);
}
}
}
```
在上述代码中,原数组`arr`包含了字符串元素`"apple"、"banana"、"orange"、"lemon"`,需要删除的目标元素为`"orange"`。将删除后的新数组存储在`newArr`中,并通过遍历`newArr`来打印删除元素后的结果。
运行结果为:
```
apple
banana
lemon
```
这样,我们就成功地删除了指定元素`"orange"`。
### 回答3:
要删除一个字符串数组中的指定元素,可以使用循环遍历数组,使用if语句判断元素是否需要删除,再通过数组拷贝的方式将不需要删除的元素放入新的数组中。
首先,我们需要创建一个新的字符串数组来存放不需要删除的元素。然后,使用for循环遍历原始字符串数组,判断当前元素是否需要删除。如果需要删除,则跳过该元素,将下一个元素放入新数组。如果不需要删除,则将该元素放入新数组中。
代码示例:
```java
public class Main {
public static void main(String[] args) {
String[] arr = {"apple", "banana", "orange", "kiwi", "watermelon"};
String target = "banana"; // 需要删除的元素
// 计算新数组的长度
int count = 0;
for (String str : arr) {
if (!str.equals(target)) {
count++;
}
}
// 创建新的字符串数组
String[] newArr = new String[count];
int index = 0;
for (String str : arr) {
if (!str.equals(target)) {
newArr[index++] = str;
}
}
// 输出新的字符串数组
for (String str : newArr) {
System.out.println(str);
}
}
}
```
上述代码中,我们创建了一个原始字符串数组arr,其中包含了一些水果名称。然后指定需要删除的元素为"banana"。程序会遍历原数组,并将不需要删除的元素复制到新的数组中。最后,输出这个新的字符串数组中的元素。
执行上述代码,输出结果为:
```
apple
orange
kiwi
watermelon
```
这样就成功地从字符串数组中删除了指定的元素"banana"。
阅读全文
相关推荐
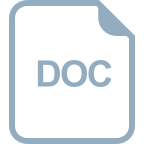
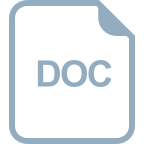
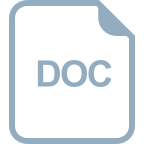

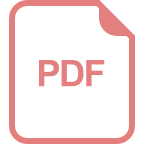
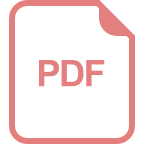
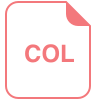
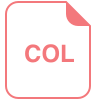
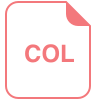
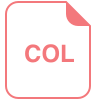






