uniapp写一个搜索组件代码
时间: 2023-09-09 13:05:09 浏览: 112
### 回答1:
可以参考下面的代码:import {$wuxSearchBar} from 'wux-weapp/dist/index'Page({
data: {
searchValue: '',
},
onLoad() {},
onReady() {},
onShow() {},
onHide() {},
onUnload() {},
onPullDownRefresh() {},
onReachBottom() {},
onShareAppMessage() {},
searchBarChange(e) {
this.setData({
searchValue: e.detail.value,
})
},
searchBarClear(e) {
this.setData({
searchValue: '',
})
},
searchBarConfirm(e) {
console.log('confirm', e.detail.value)
},
components: {
$wuxSearchBar
}
})
### 回答2:
Uniapp是一个基于Vue.js框架的跨平台开发工具,可以使用Vue语法编写组件。下面是一个简单的搜索组件的代码示例:
```html
<template>
<view class="search">
<input type="text" v-model="keyword" @change="search" placeholder="请输入关键字" />
<button @click="search">搜索</button>
<view v-if="result.length">
<view v-for="(item, index) in result" :key="index" class="item">
<text>{{ item }}</text>
</view>
</view>
<view v-else class="empty">
<text>暂无结果</text>
</view>
</view>
</template>
<script>
export default {
data() {
return {
keyword: '', // 搜索关键字
result: [] // 搜索结果
};
},
methods: {
search() {
// 发送搜索请求,获取结果
// 假设请求接口返回结果为数组
// 实际项目中需要使用uni.request或axios等发送网络请求
// 并处理返回的数据
this.result = [
'搜索结果1',
'搜索结果2',
'搜索结果3'
];
}
}
};
</script>
<style>
.search {
margin: 20px;
}
.item {
padding: 10px;
border: 1px solid #ccc;
margin-bottom: 10px;
}
.empty {
text-align: center;
margin-top: 20px;
}
</style>
```
以上代码实现了一个简单的搜索组件。用户可以在输入框中输入关键字并点击搜索按钮,组件会根据关键字发送搜索请求,并显示返回的搜索结果。如果搜索结果为空,会显示"暂无结果"。
### 回答3:
uniapp是一种基于Vue开发的跨端框架,可以同时开发微信小程序、H5、安卓和iOS应用。为了编写一个搜索组件,首先需要在uniapp项目中创建一个组件文件夹,比如命名为Search。
在Search文件夹中,我们创建三个文件:Search.vue、Search.js和Search.json。
在Search.vue中,我们编写组件的模板和样式,如下所示:
<template>
<div class="search">
<input
type="text"
v-model="keyword"
@input="handleInput"
placeholder="请输入关键字"
/>
<button @click="handleSearch">搜索</button>
</div>
</template>
<script>
export default {
data() {
return {
keyword: ""
};
},
methods: {
handleInput(event) {
this.keyword = event.target.value;
},
handleSearch() {
this.$emit("search", this.keyword);
}
}
};
</script>
<style scoped>
.search {
display: flex;
align-items: center;
}
input {
flex: 1;
height: 40px;
padding: 0 10px;
border: 1px solid #ccc;
border-right: none;
}
button {
height: 40px;
}
</style>
在Search.js中,我们可以编写一些组件逻辑,例如处理搜索事件的回调函数。可以根据实际需求进行编写。
在Search.json中,我们可以配置组件的一些属性和样式。可以根据实际需求进行配置。
最后,在使用Search组件的页面中,引入Search组件并使用它,如下所示:
<template>
<div>
<search @search="handleSearch" />
</div>
</template>
<script>
import Search from "@/components/Search/Search.vue";
export default {
components: {
Search
},
methods: {
handleSearch(keyword) {
// 处理搜索事件
console.log(keyword);
}
}
};
</script>
这样就完成了一个简单的搜索组件的编写。用户可以在输入框中输入关键字,然后点击搜索按钮,通过回调函数将关键字传递给父组件进行处理。
阅读全文
相关推荐
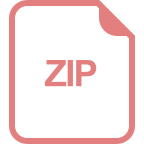
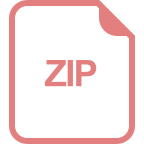


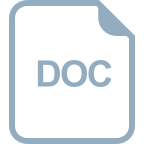












