给tablayout的每个tab设置边框,且边框与边框之间有间距
时间: 2023-11-22 15:56:02 浏览: 35
可以使用自定义View来实现该效果,具体步骤如下:
1. 创建一个自定义的TabLayout,继承自TabLayout。
2. 重写TabLayout的onDraw方法,在该方法中绘制每个tab的边框。
3. 在onMeasure方法中,计算每个tab的宽度,并添加间距。
4. 在TabLayout的addTab方法中,为每个Tab设置自定义的View。
以下是示例代码,供参考:
```java
public class CustomTabLayout extends TabLayout {
private Paint mPaint;
private int mIndicatorColor = Color.RED;
private int mSelectedPosition = 0;
private int mIndicatorHeight = 5;
private int mTabPadding = 20;
private int mTabSpacing = 20;
public CustomTabLayout(Context context) {
super(context);
init();
}
public CustomTabLayout(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public CustomTabLayout(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
mPaint = new Paint();
mPaint.setAntiAlias(true);
mPaint.setStyle(Paint.Style.FILL);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
// 绘制tab的边框
mPaint.setColor(mIndicatorColor);
mPaint.setStrokeWidth(mIndicatorHeight);
int tabCount = getTabCount();
for (int i = 0; i < tabCount; i++) {
View tabView = getTabAt(i).getCustomView();
if (tabView != null) {
int left = tabView.getLeft();
int right = tabView.getRight();
int top = tabView.getTop();
int bottom = tabView.getBottom();
int centerX = (left + right) / 2;
if (i == mSelectedPosition) {
canvas.drawLine(left, bottom, right, bottom, mPaint);
} else {
canvas.drawLine(left, bottom, centerX - mTabSpacing / 2, bottom, mPaint);
canvas.drawLine(centerX + mTabSpacing / 2, bottom, right, bottom, mPaint);
}
}
}
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
// 计算每个tab的宽度,并添加间距
int tabCount = getTabCount();
for (int i = 0; i < tabCount; i++) {
View tabView = getTabAt(i).getCustomView();
if (tabView != null) {
TextView tabTextView = tabView.findViewById(android.R.id.text1);
tabTextView.setPadding(mTabPadding, 0, mTabPadding, 0);
int width = tabTextView.getMeasuredWidth() + mTabPadding * 2 + mTabSpacing;
tabView.setMinimumWidth(width);
}
}
}
@Override
public void addTab(@NonNull Tab tab) {
super.addTab(tab);
// 为每个Tab设置自定义的View
View tabView = LayoutInflater.from(getContext()).inflate(R.layout.custom_tab, null);
TextView tabTextView = tabView.findViewById(android.R.id.text1);
tabTextView.setText(tab.getText());
tab.setCustomView(tabView);
}
public void setSelectedPosition(int position) {
mSelectedPosition = position;
invalidate();
}
}
```
其中,自定义的TabLayout中使用了R.layout.custom_tab布局文件来设置每个tab的样式,该布局文件可以根据实际需求自行定义。在该布局文件中,需要使用android.R.id.text1来设置tab的文本。
示例布局文件如下:
```xml
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/text1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@color/tab_text_color"
android:textSize="@dimen/tab_text_size"
android:textStyle="bold" />
```
在使用该自定义TabLayout时,只需要在xml文件中引用即可:
```xml
<com.example.CustomTabLayout
android:id="@+id/tabLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:tabIndicatorColor="@color/tab_indicator_color"
app:tabSelectedTextColor="@color/tab_text_color"
app:tabTextAppearance="@style/CustomTabTextAppearance"
app:tabTextColor="@color/tab_text_color" />
```
其中,app:tabIndicatorColor、app:tabSelectedTextColor、app:tabTextAppearance、app:tabTextColor等属性与普通TabLayout使用方法一致。
相关推荐
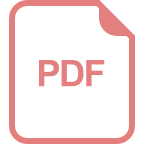
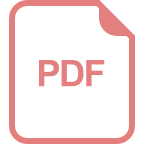
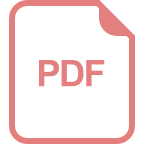














