netty demo
时间: 2023-08-02 11:12:18 浏览: 129
以下是一个简单的 Netty 服务器和客户端的示例代码:
服务端:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFutureListener;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
import io.netty.util.CharsetUtil;
public class NettyServerDemo {
private static final int PORT = 8080;
public static void main(String[] args) {
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(new NioEventLoopGroup(), new NioEventLoopGroup())
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new StringDecoder(CharsetUtil.UTF_8));
pipeline.addLast(new StringEncoder(CharsetUtil.UTF_8));
pipeline.addLast(new SimpleChannelInboundHandler<String>() {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println("Server received: " + msg);
ctx.writeAndFlush("Hello from server!");
}
});
}
});
Channel channel = serverBootstrap.bind(PORT).syncUninterruptibly().channel();
System.out.println("Server started on port " + PORT);
channel.closeFuture().addListener((ChannelFutureListener) future -> {
System.out.println("Server stopped.");
});
}
}
```
客户端:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFutureListener;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
import io.netty.util.CharsetUtil;
import java.util.Scanner;
public class NettyClientDemo {
private static final String HOST = "localhost";
private static final int PORT = 8080;
public static void main(String[] args) {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(new NioEventLoopGroup())
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new StringDecoder(CharsetUtil.UTF_8));
pipeline.addLast(new StringEncoder(CharsetUtil.UTF_8));
pipeline.addLast(new SimpleChannelInboundHandler<String>() {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println("Client received: " + msg);
}
});
}
});
Channel channel = bootstrap.connect(HOST, PORT).syncUninterruptibly().channel();
System.out.println("Client connected to server on " + HOST + ":" + PORT);
Scanner scanner = new Scanner(System.in);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
if ("exit".equals(line)) {
break;
}
channel.writeAndFlush(line);
}
channel.close().addListener((ChannelFutureListener) future -> {
System.out.println("Client disconnected from server.");
});
}
}
```
这个示例代码实现了一个简单的文本协议,客户端输入的所有内容都会发送到服务端,服务端会将收到的消息原样返回给客户端。你可以基于这个示例代码构建更复杂的网络应用。
阅读全文
相关推荐
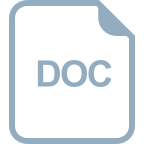
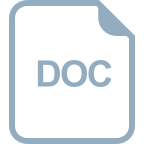
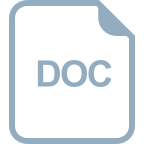
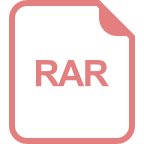
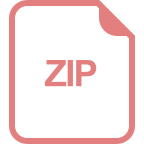
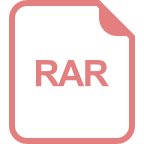
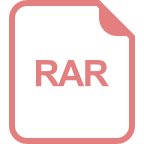
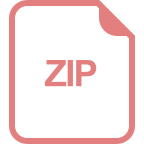
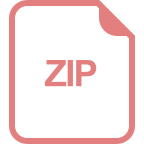
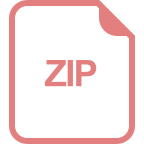
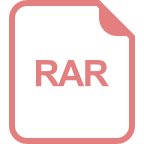
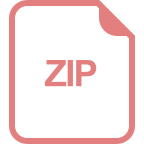

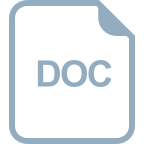
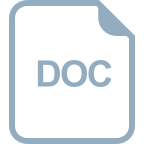
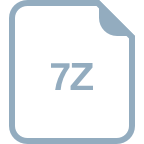
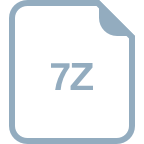