用python写一个猜拳游戏
时间: 2023-09-09 10:05:09 浏览: 63
### 回答1:
好的,这是一个简单的猜拳游戏程序:
```
import random
# 定义可能出现的选项
options = ['剪刀', '石头', '布']
# 让用户输入选项
user_choice = input('请出拳(剪刀/石头/布): ')
# 让电脑随机出拳
computer_choice = random.choice(options)
# 判断输赢
if user_choice == computer_choice:
print('平局')
elif user_choice == '剪刀' and computer_choice == '布':
print('你赢了')
elif user_choice == '石头' and computer_choice == '剪刀':
print('你赢了')
elif user_choice == '布' and computer_choice == '石头':
print('你赢了')
else:
print('你输了')
# 输出电脑的出拳
print(f'电脑出拳: {computer_choice}')
```
这个程序会让用户输入一个选项,然后让电脑随机出拳。最后根据猜拳的规则判断输赢,并输出结果。
### 回答2:
下面是一个用Python编写的猜拳游戏的示例代码:
```python
import random
def get_user_choice():
"""获取用户的猜拳选择"""
while True:
choice = input("请选择(剪刀/石头/布):")
if choice.lower() in ['剪刀', '石头', '布']:
return choice.lower()
else:
print("输入无效,请重新选择。")
def get_computer_choice():
"""获取电脑的随机猜拳选择"""
choices = ['剪刀', '石头', '布']
return random.choice(choices)
def determine_winner(user_choice, computer_choice):
"""判断获胜者"""
if user_choice == computer_choice:
return "平局"
elif (user_choice == '剪刀' and computer_choice == '布') or (user_choice == '石头' and computer_choice == '剪刀') or (user_choice == '布' and computer_choice == '石头'):
return "用户胜利"
else:
return "电脑胜利"
def play_game():
"""玩一局猜拳游戏"""
user_choice = get_user_choice()
computer_choice = get_computer_choice()
print("用户选择:" + user_choice)
print("电脑选择:" + computer_choice)
winner = determine_winner(user_choice, computer_choice)
print("结果:" + winner)
def main():
"""主函数"""
play_again = True
while play_again:
play_game()
choice = input("是否继续游戏?(是/否):")
if choice.lower() == '否':
play_again = False
# 启动游戏
main()
```
这是一个简单的猜拳游戏,玩家可以选择剪刀、石头或布,电脑随机产生一个选择,然后根据规则判断胜负。游戏结束后,玩家可以选择是否继续游戏。
相关推荐
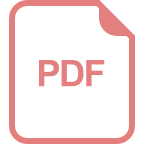
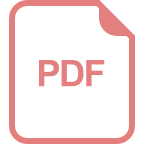
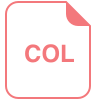
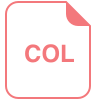
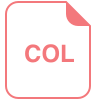
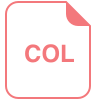
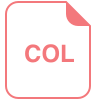








