c语言用do while求500以内的全部素数,每行输出十个
时间: 2024-01-06 16:04:52 浏览: 33
```
#include <stdio.h>
int main() {
int i, j, flag, count;
i = 2; // 从2开始判断
count = 0; // 计数器,每行输出十个
do {
flag = 1; // 假设i是素数
for (j = 2; j < i; j++) {
if (i % j == 0) { // i能被j整除,不是素数
flag = 0; // 修改标志
break; // 跳出循环
}
}
if (flag) { // 如果i是素数
printf("%d ", i); // 输出i
count++; // 计数器加1
if (count == 10) { // 如果已经输出了十个
printf("\n"); // 换行
count = 0; // 计数器清零
}
}
i++; // 判断下一个数
} while (i <= 500); // 判断500以内的数
return 0;
}
```
相关问题
求500以内的全部素数,每行输出10个do while语句
int num = 2;
do {
int i = 2;
bool isPrime = true;
while (i <= sqrt(num)) {
if (num % i == 0) {
isPrime = false;
break;
}
i++;
}
if (isPrime) {
cout << num << " ";
if (num % 10 == 1) {
cout << endl;
}
}
num++;
} while (num <= 500);
用C语言求100以内的全部素数,每行输出10个
以下是用C语言编写的求100以内全部素数的程序,每行输出10个:
```c
#include <stdio.h>
int main() {
int i, j, flag;
for (i = 2; i <= 100; i++) {
flag = 1;
for (j = 2; j < i; j++) {
if (i % j == 0) {
flag = 0;
break;
}
}
if (flag) {
printf("%d ", i);
if (i % 10 == 1) {
printf("\n");
}
}
}
return 0;
}
```
程序中使用了两个循环,外层循环从2到100遍历每一个数字,内层循环从2到当前数字的前一个数字,判断当前数字是否为素数。如果当前数字能够被内层循环中的任意一个数字整除,则不是素数,标志位flag被设置为0,内层循环结束。如果内层循环结束后flag仍然为1,则当前数字是素数,输出并判断是否需要换行。
相关推荐
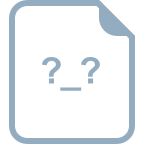












