python滑动相关算法
时间: 2023-06-18 22:07:24 浏览: 146
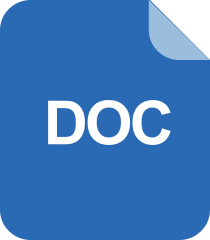
滑动窗口算法

Python中常用的滑动相关算法有以下几种:
1. 滑动窗口
滑动窗口算法是一种常见的算法,用于解决字符串和数组的子串或子序列问题。其基本思想是维护一个窗口,通过移动窗口的起始和结束位置,来寻找符合条件的子串或子序列。
示例代码:
```python
def sliding_window(nums, k):
if not nums:
return []
n = len(nums)
res = []
l, r = 0, 0
while r < n:
# 移动右指针
while r < n and r - l < k:
r += 1
# 判断是否符合条件
if r - l == k:
res.append(nums[l:r])
# 移动左指针
l += 1
return res
```
2. 双指针
双指针算法也是一种常见的算法,用于解决数组或链表中的问题。其基本思想是维护两个指针,分别指向数组或链表的不同位置,通过移动指针来寻找符合条件的元素。
示例代码:
```python
def two_pointers(nums, target):
if not nums:
return []
n = len(nums)
res = []
i, j = 0, n - 1
while i < j:
if nums[i] + nums[j] == target:
res.append([nums[i], nums[j]])
i += 1
j -= 1
elif nums[i] + nums[j] < target:
i += 1
else:
j -= 1
return res
```
3. 滑动窗口+双指针
滑动窗口+双指针算法是一种结合了上述两种算法的算法,用于解决数组或字符串中的问题。其基本思想是维护一个窗口和两个指针,通过移动窗口和指针来寻找符合条件的元素。
示例代码:
```python
def sliding_window_and_two_pointers(nums, target):
if not nums:
return []
n = len(nums)
res = []
l, r = 0, 1
while l < n:
# 移动右指针
while r < n and nums[r] - nums[l] <= target:
if nums[r] - nums[l] == target:
res.append([nums[l], nums[r]])
r += 1
# 移动左指针
l += 1
return res
```
以上是三种常见的滑动相关算法,它们在不同的场景下都有着重要的应用。
阅读全文
相关推荐


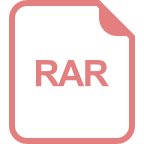
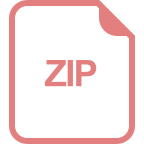










