使用AVFoundation框架开发一个能拍照并保存照片到相册的iOS app demo
时间: 2024-02-29 07:51:30 浏览: 24
好的,以下是使用 AVFoundation 框架开发一个能拍照并保存照片到相册的 iOS app demo 的步骤:
1. 创建一个新的 Xcode 项目,选择 Single View App 模板,并勾选 "Use SwiftUI" 选项。
2. 在 ContentView.swift 文件中,创建一个 preview 块,并添加一个 Button 视图。
3. 在 Button 的 action 中,调用一个名为 takePhoto 的函数。
4. 在 ContentView.swift 文件中,创建一个名为 CameraView 的自定义视图,并在其中导入 AVFoundation 框架。
5. 在 CameraView 中添加 AVCaptureSession、AVCapturePhotoOutput 和 AVCaptureVideoPreviewLayer 属性。
6. 在 CameraView 的 init 方法中,配置 AVCaptureSession,并将 AVCaptureVideoPreviewLayer 添加到视图上。
7. 实现 takePhoto 函数,在其中调用 AVCapturePhotoOutput 的 capturePhoto 方法,并在 completionHandler 中将照片保存到相册中。
8. 在 ContentView 中,将 CameraView 添加为一个子视图,并设置其大小和位置。
9. 运行项目,在模拟器或真机上测试拍照和保存照片的功能。
以下是示例代码:
```swift
import SwiftUI
import AVFoundation
import Photos
struct ContentView: View {
var body: some View {
VStack {
CameraView()
.frame(width: 300, height: 300)
Button("Take Photo") {
takePhoto()
}
}
}
func takePhoto() {
// TODO: Call takePhoto function in CameraView
}
}
struct CameraView: UIViewRepresentable {
private let captureSession = AVCaptureSession()
private let photoOutput = AVCapturePhotoOutput()
private let videoPreviewLayer = AVCaptureVideoPreviewLayer()
func makeUIView(context: Context) -> UIView {
let view = UIView(frame: .zero)
configureCaptureSession()
videoPreviewLayer.frame = view.layer.bounds
view.layer.addSublayer(videoPreviewLayer)
captureSession.startRunning()
return view
}
func updateUIView(_ uiView: UIView, context: Context) {
videoPreviewLayer.frame = uiView.layer.bounds
}
func configureCaptureSession() {
guard let device = AVCaptureDevice.default(for: .video),
let input = try? AVCaptureDeviceInput(device: device) else {
return
}
if captureSession.canAddInput(input) {
captureSession.addInput(input)
}
if captureSession.canAddOutput(photoOutput) {
captureSession.addOutput(photoOutput)
}
videoPreviewLayer.session = captureSession
}
func takePhoto() {
let settings = AVCapturePhotoSettings()
photoOutput.capturePhoto(with: settings, delegate: self)
}
}
extension CameraView: AVCapturePhotoCaptureDelegate {
func photoOutput(_ output: AVCapturePhotoOutput, didFinishProcessingPhoto photo: AVCapturePhoto, error: Error?) {
guard let imageData = photo.fileDataRepresentation(),
let image = UIImage(data: imageData) else {
return
}
PHPhotoLibrary.shared().performChanges({
PHAssetChangeRequest.creationRequestForAsset(from: image)
}) { (success, error) in
if success {
print("Photo saved to library")
} else if let error = error {
print("Error saving photo to library: \(error.localizedDescription)")
}
}
}
}
```
注意:在运行项目之前,需要在 info.plist 文件中添加 "Privacy - Camera Usage Description" 和 "Privacy - Photo Library Additions Usage Description" 权限描述。
相关推荐
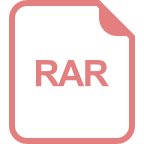
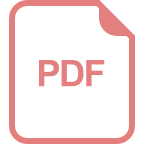














