java使用接口和抽象函类进行写1.编写程序:在教学管理系统中有教师、教学管理人员和学生,定义一个抽象类 Person,用 Teacher、 Manager、 Student去继承 Person类,完成类的设计.
时间: 2024-02-06 21:11:47 浏览: 74
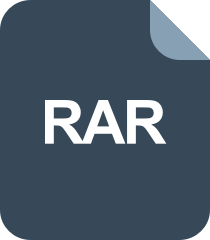
Experiment.rar
以下是使用接口和抽象类进行实现的 Java 程序:
```java
// 定义 Person 抽象类
public abstract class Person {
protected String name;
protected int age;
protected String gender;
public Person(String name, int age, String gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
public abstract void introduce();
}
// 定义 IPerson 接口
public interface IPerson {
void introduce();
}
// 定义 Teacher 类,实现 IPerson 接口
public class Teacher extends Person implements IPerson {
private String department;
public Teacher(String name, int age, String gender, String department) {
super(name, age, gender);
this.department = department;
}
@Override
public void introduce() {
System.out.printf("I am a teacher. My name is %s, and I am %d years old. I work in the %s department.\n", name, age, department);
}
}
// 定义 Manager 类,实现 IPerson 接口
public class Manager extends Person implements IPerson {
private String title;
public Manager(String name, int age, String gender, String title) {
super(name, age, gender);
this.title = title;
}
@Override
public void introduce() {
System.out.printf("I am a manager. My name is %s, and I am %d years old. My title is %s.\n", name, age, title);
}
}
// 定义 Student 类,实现 IPerson 接口
public class Student extends Person implements IPerson {
private int grade;
public Student(String name, int age, String gender, int grade) {
super(name, age, gender);
this.grade = grade;
}
@Override
public void introduce() {
System.out.printf("I am a student. My name is %s, and I am %d years old. I am in grade %d.\n", name, age, grade);
}
}
```
在这个程序中,我们定义了一个抽象类 `Person`,其中包含三个属性 `name`、`age` 和 `gender`,以及一个抽象方法 `introduce`。我们还定义了一个接口 `IPerson`,包含一个抽象方法 `introduce`。然后,我们定义了 `Teacher`、`Manager` 和 `Student` 三个类,它们都继承自 `Person` 类,并实现了 `IPerson` 接口中的 `introduce` 方法。
我们可以使用这个程序,创建不同类型的人物对象:
```java
Teacher t = new Teacher("Tom", 35, "male", "mathematics");
t.introduce(); // 输出:I am a teacher. My name is Tom, and I am 35 years old. I work in the mathematics department.
Manager m = new Manager("Mary", 40, "female", "dean");
m.introduce(); // 输出:I am a manager. My name is Mary, and I am 40 years old. My title is dean.
Student s = new Student("Sam", 18, "male", 12);
s.introduce(); // 输出:I am a student. My name is Sam, and I am 18 years old. I am in grade 12.
```
这样,我们就使用接口和抽象类完成了题目中要求的类的设计。
阅读全文
相关推荐
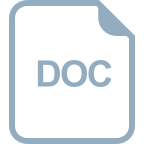
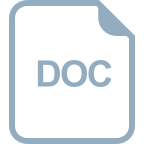
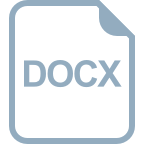
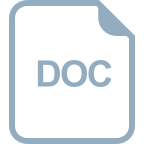
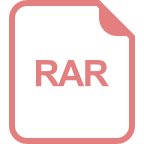
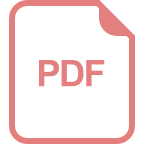
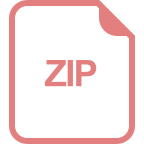
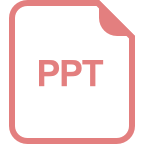
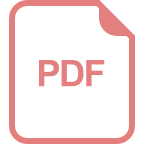
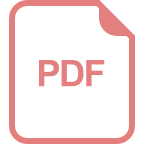
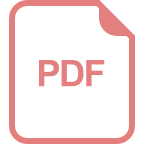
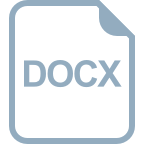
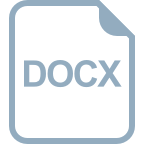
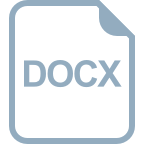
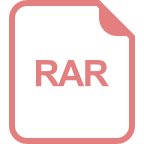
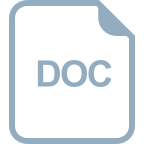
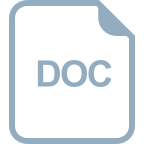
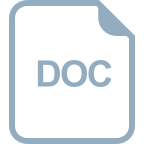