根据最上方代码 解释下 while node: print(node.val, end=' ') node = node.next 中while循环的用法
时间: 2024-04-14 08:31:30 浏览: 57
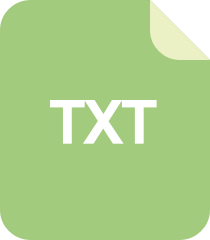
node.js redis资料

在上方的代码中,`while node:` 是一个循环结构,其作用是在链表中遍历节点并打印节点的值。
首先,我们初始化一个变量 `node`,并将其赋值为链表的头节点 `head`。然后,我们使用 `while` 循环来判断 `node` 是否存在(即非空),如果存在则执行循环体内的代码。
循环体内的代码是 `print(node.val, end=' ')` 和 `node = node.next`。`print(node.val, end=' ')` 表示打印当前节点的值,并且使用 `end=' '` 参数设置输出末尾不换行,而是以空格结尾。然后,我们将 `node` 更新为下一个节点,即 `node.next`,以便在下一次循环中处理下一个节点。
通过这样的方式,循环会一直执行直到链表中的所有节点都被遍历完毕(即 `node` 变为 `None`)为止。
总结起来,`while node:` 循环的作用是遍历链表中的节点,并对每个节点执行一些操作,例如打印节点的值或修改节点的属性。
希望这个解释对你有帮助!
阅读全文
相关推荐
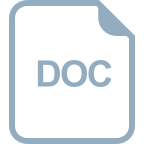
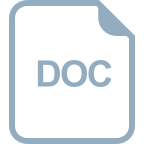

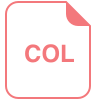
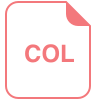
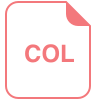
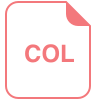
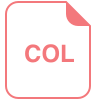
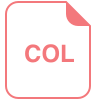








