编写程序,检查字符串" life is short. i use python"中是否包含字符串"python",若包含则替换为"python"后输出新字符串,否则输出原字符串。
时间: 2023-05-31 16:20:11 浏览: 952
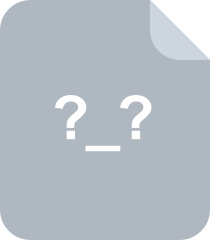
python 写一个函数,求一个字符串的长度,在main函数中输入字符串,并输出其长度(示例)
### 回答1:
可以使用Python中的字符串方法find()来检查字符串是否包含另一个字符串,如果返回值不为-1,则说明包含,可以使用replace()方法进行替换,否则输出原字符串。
代码如下:
```python
s = "life is short. i use python"
if s.find("python") != -1:
s = s.replace("python", "Python")
print(s)
else:
print(s)
```
输出结果为:
```
life is short. i use Python
```
### 回答2:
这个问题很简单,我们可以使用 Python 内置的字符串方法来找到目标字符串是否在给定的字符串中出现过,然后根据结果进行字符串替换或输出原字符串。
以下是 Python 实现代码:
```python
# 定义要检查的字符串
string = "life is short. i use python"
# 判断字符串中是否包含目标字符串
if "python" in string:
# 如果包含目标字符串,则进行替换,并输出新字符串
new_string = string.replace("python", "Python")
print("替换后的字符串为:{}".format(new_string))
else:
# 如果不包含目标字符串,直接输出原字符串
print("原字符串为:{}".format(string))
```
上述代码中,我们首先定义了要检查的字符串,然后使用 `in` 关键字判断目标字符串 `"python"` 是否在字符串中出现过。如果出现过,则使用 `replace` 方法将 `"python"` 替换为 `"Python"`,并将新字符串打印出来;否则,直接将原字符串打印出来。
需要注意的是,上述代码中的字符串替换使用了 `replace` 方法,该方法会返回一个新字符串,原字符串并不会改变。如果我们想在原字符串中直接替换,可以使用字符串操作符 `+=`。例如:
```python
# 定义要检查的字符串
string = "life is short. i use python"
# 判断字符串中是否包含目标字符串
if "python" in string:
# 如果包含目标字符串,则进行替换
string = string.replace("python", "Python")
# 直接输出原字符串,此时已经替换完成
print("替换后的字符串为:{}".format(string))
```
上述代码中,我们使用 `replace` 方法将 `"python"` 替换为 `"Python"`,并将替换后的新字符串直接赋值给原字符串 `string`。这样,原字符串就会被直接替换。然后,我们直接输出替换后的原字符串。
### 回答3:
要编写程序来检查字符串"life is short. i use python"中是否包含字符串"python",并在包含的情况下将"python"替换为"Python"后输出新字符串,否则输出原字符串。这个问题可以使用Python的字符串操作来解决。
我们可以使用Python的字符串查找方法find()来检查原始字符串中是否存在子字符串"python"。如果find()方法返回的是-1,则表示子字符串不存在,否则返回子字符串的索引位置。如果find()方法返回的索引位置是大于等于0的,则表示子字符串存在,可以使用replace()方法来替换该子字符串。
下面是一个简单的程序来解决这个问题:
```
str = "life is short. i use python"
# 检查子字符串是否存在
if str.find("python") >= 0:
# 替换子字符串为"Python"
new_str = str.replace("python", "Python")
print(new_str)
else:
print(str)
```
在上述程序中,我们首先定义了原始字符串str。然后使用if语句检查子字符串是否存在于原始字符串中,如果存在,则使用replace()方法替换它为"Python",并将新字符串赋值给变量new_str。最后,程序使用print()语句来输出新字符串或原始字符串,具体取决于子字符串的存在与否。
运行上述程序将输出"life is short. i use Python",因为它包含子字符串"python"。如果原始字符串不包含子字符串"python",则程序将输出原始字符串"life is short. i use python"。
阅读全文
相关推荐
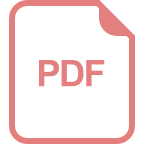
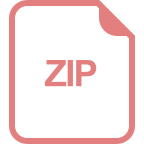















