用一个子函数一个主函数用c语言写该函数可以统计字符串中的数字字符个数,并作为函数值返回。
时间: 2024-03-11 11:48:53 浏览: 52
可以使用以下代码实现该函数:
```c
#include <stdio.h>
int count_digits(char *str);
int main() {
char str[100];
printf("Enter a string: ");
scanf("%s", str);
int num_digits = count_digits(str);
printf("Number of digits in the string: %d\n", num_digits);
return 0;
}
int count_digits(char *str) {
int count = 0;
while (*str) {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
```
这个程序包含了一个名为 `count_digits` 的子函数和一个名为 `main` 的主函数。主函数首先提示用户输入一个字符串,并将该字符串存储在一个字符数组中。然后它调用 `count_digits` 函数来计算字符串中的数字字符个数,并将计数结果打印出来。
`count_digits` 函数的实现与上一个问题中的实现相同。它遍历字符串中的每个字符,如果该字符是数字字符,则将计数器加一。最后,该函数返回计数器的值,即数字字符的个数。
相关问题
用c语言的复合函数写该函数可以统计字符串中的数字字符个数,并作为函数值返回。
可以使用以下的代码实现该函数:
```c
int count_digits(char *str) {
int count = 0;
while (*str) {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
```
这个函数的实现比较简单。它使用一个 while 循环遍历字符串中的每个字符,如果该字符是数字字符,则将计数器加一。最后,该函数返回计数器的值,即数字字符的个数。
c语言编写函数fun,统计s所指字符串中的数字字符个数,并作为函数值返回
可以使用以下代码编写函数fun来实现统计字符串中数字字符的个数:
```c
int fun(char *s){
int count = 0;
while(*s != '\0'){
if(*s >= '0' && *s <= '9'){
count++;
}
s++;
}
return count;
}
```
在上面的代码中,我们使用一个指针 `s` 来遍历字符串中的每个字符,如果当前字符是数字字符,则计数器 `count` 加一。遍历结束后,将计数器的值作为函数返回值返回即可。
注意,上面的代码中使用了指针遍历字符串,因此需要确保指针 `s` 所指向的字符串不为空,并以空字符('\0')结尾。
相关推荐
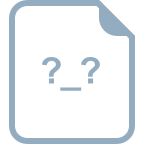












