python合并列表中的字典
时间: 2023-12-29 20:56:46 浏览: 23
可以使用 Python 的 `collections` 模块中的 `ChainMap` 类来合并列表中的字典。例如:
```
from collections import ChainMap
dict_list = [{'a': 1, 'b': 2}, {'c': 3, 'd': 4}, {'e': 5, 'f': 6}]
merged_dict = dict(ChainMap(*dict_list))
print(merged_dict)
```
输出结果为:
```
{'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5, 'f': 6}
```
也可以使用 python3.5+的语法,使用 **字典解析**
```
dict_list = [{'a': 1, 'b': 2}, {'c': 3, 'd': 4}, {'e': 5, 'f': 6}]
merged_dict = {k: v for d in dict_list for k, v in d.items()}
print(merged_dict)
```
这两种方法都能得到一个新的字典,其中包含了列表中所有字典的键值对。
相关问题
python将列表中字典的键值合并
假设你有一个列表,其中包含多个字典,每个字典都有相同的键,你可以使用一个循环遍历列表中的每个字典,将其键值合并到一个新的字典中。以下是一个示例代码:
```python
my_list = [{'name': 'Alice', 'age': 25, 'city': 'New York'},
{'name': 'Bob', 'age': 30, 'city': 'Los Angeles'},
{'name': 'Charlie', 'age': 35, 'city': 'Chicago'}]
merged_dict = {}
for my_dict in my_list:
for key, value in my_dict.items():
merged_dict.setdefault(key, []).append(value)
print(merged_dict)
```
输出结果为:`{'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35], 'city': ['New York', 'Los Angeles', 'Chicago']}`
在上面的代码中,我们首先定义了一个空字典 `merged_dict` 用来存储合并后的键值对。然后我们使用两个嵌套的循环,首先遍历列表中的每个字典,然后再遍历每个字典中的键值对。对于每个键值对,我们将键作为新字典的键,并将值追加到字典中已有的值列表中(如果该键还不存在于字典中,则创建一个新的值列表)。最后,我们打印出合并后的字典 `merged_dict`。
python 两个列表中字典合并
可以使用列表解析和字典的update()方法来合并两个列表中的字典。假设有两个列表list1和list2,每个列表中包含多个字典,可以使用以下代码将它们合并:
```python
merged_list = [dict1.update(dict2) or dict1 for dict1, dict2 in zip(list1, list2)]
```
这里使用了zip()函数来同时迭代两个列表中的字典,然后将每个字典的update()方法应用于另一个字典。注意,update()方法会修改原始字典,并且返回值为None,因此需要使用or运算符将修改后的字典作为结果返回。
如果要将两个列表中的所有字典合并为一个新的字典,可以使用以下代码:
```python
merged_dict = {}
for dict1 in list1 + list2:
merged_dict.update(dict1)
```
这里将两个列表连接为一个新的列表,然后迭代该列表中的每个字典并将其update()方法应用于merged_dict字典。最终得到的merged_dict字典包含了所有的键值对。
相关推荐
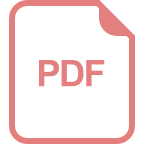
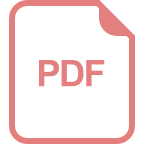
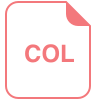
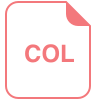
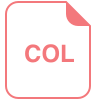
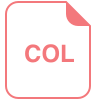
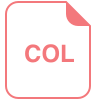







