1.对数据进行处理(删除空白值,重复值等) 2.在不选择特征(不降维的情况下),利用LogisticsRegression、RandomForest、SVM 对数据进行建模。 3.利用PAC或者随机森林的特征重要性分析选择属性,达到降维的目的。 4.在确定重要属性后,再次构建模型,对比模型之间的差异。 5.最后得到关于适合本数据的模型,并可对未来数据进行预测,并为医院管理人员提出相关的建议这个思路的PP咋做
时间: 2024-02-18 08:06:19 浏览: 16
以下是一个基本的代码示例,演示如何执行上述操作。请注意,这只是一个示例,您需要根据您的数据集进行适当的修改和调整。
```python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.ensemble import RandomForestClassifier
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score, classification_report
from sklearn.feature_selection import SelectFromModel
from sklearn.ensemble import ExtraTreesClassifier
# 读取数据
data = pd.read_csv('data.csv')
# 删除空白值和重复值
data = data.dropna()
data = data.drop_duplicates()
# 划分数据集
X = data.drop('target', axis=1)
y = data['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 建立模型
models = [LogisticRegression(), RandomForestClassifier(), SVC()]
for model in models:
# 不选择特征,使用原始数据建模
print(type(model).__name__, '--- without feature selection ---')
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
print(classification_report(y_test, y_pred, target_names=['class 0', 'class 1']))
# 使用特征重要性分析进行特征选择
if type(model).__name__ != 'SVC':
print(type(model).__name__, '--- with feature selection ---')
clf = ExtraTreesClassifier()
clf.fit(X_train, y_train)
feature_importances = pd.DataFrame(clf.feature_importances_, index=X_train.columns, columns=['importance'])
feature_importances.sort_values(by='importance', ascending=False, inplace=True)
print(feature_importances)
sfm = SelectFromModel(clf, threshold='mean')
sfm.fit(X_train, y_train)
X_train_selected = sfm.transform(X_train)
X_test_selected = sfm.transform(X_test)
model.fit(X_train_selected, y_train)
y_pred = model.predict(X_test_selected)
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
print(classification_report(y_test, y_pred, target_names=['class 0', 'class 1']))
```
此代码将:
1. 读取数据并删除空白值和重复值。
2. 将数据集划分为训练集和测试集。
3. 使用逻辑回归、随机森林和支持向量机等模型对原始数据进行建模,并计算精度和分类报告。
4. 对于每个模型,使用特征重要性分析进行特征选择,使用选定的特征重新建立模型,并计算精度和分类报告。
您可以根据需要修改此代码,例如,您可能需要对数据进行缩放或进行其他预处理步骤,或者您可能需要使用其他模型进行建模。此外,您还可以通过交叉验证等技术来更准确地评估模型性能。
相关推荐
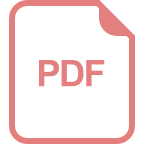














