C#实现DataTable转换为实体的方案
时间: 2024-02-05 11:11:51 浏览: 214
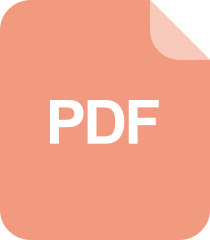
C# DataTable 转换为 实体类对象实例
在 C# 中将 DataTable 转换为实体有多种方案,下面介绍其中比较常用的两种。
1. 使用反射实现
这种方法是通过使用反射获取 DataTable 中的列名和对应的值,然后通过反射将值赋给实体对象的属性。代码如下:
```csharp
public static List<T> DataTableToList<T>(DataTable dt) where T : new()
{
List<T> list = new List<T>();
foreach (DataRow dr in dt.Rows)
{
T t = new T();
PropertyInfo[] propInfos = t.GetType().GetProperties();
foreach (PropertyInfo propInfo in propInfos)
{
if (dt.Columns.Contains(propInfo.Name))
{
object value = dr[propInfo.Name];
if (value != DBNull.Value)
{
propInfo.SetValue(t, value, null);
}
}
}
list.Add(t);
}
return list;
}
```
使用示例:
```csharp
DataTable dt = GetDataTable();
List<MyEntity> list = DataTableToList<MyEntity>(dt);
```
2. 使用泛型类库实现
这种方法是通过使用泛型类库来实现 DataTable 转换为实体,例如使用 Dapper 类库。Dapper 是一个轻量级的 ORM 类库,可以方便地将数据从数据库中读取到实体对象中。使用 Dapper 类库将 DataTable 转换为实体的代码如下:
```csharp
public static List<T> DataTableToList<T>(DataTable dt) where T : new()
{
List<T> list = new List<T>();
using (var conn = new SqlConnection(connectionString))
{
conn.Open();
list = conn.Query<T>("select * from @dt", new { dt = dt }).ToList();
}
return list;
}
```
使用示例:
```csharp
DataTable dt = GetDataTable();
List<MyEntity> list = DataTableToList<MyEntity>(dt);
```
注意:使用 Dapper 类库需要先安装 NuGet 包,在控制台中执行以下命令即可:
```bash
Install-Package Dapper
```
阅读全文
相关推荐
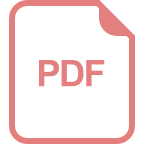
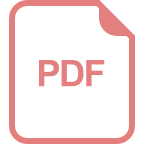
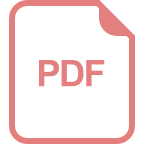
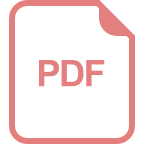
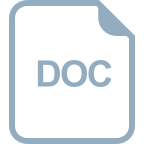
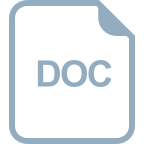




