给出二十个c++考试常考的函数,给出具体代码例子
时间: 2024-03-25 19:40:57 浏览: 62
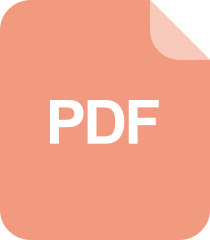
C++ Certified Professional Programmer (61-120).pdf
好的,以下是二十个C++考试常考的函数以及代码示例:
1. strlen:用于计算字符串长度
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[] = "Hello, world!";
int len = strlen(str);
cout << "The length of the string is: " << len << endl;
return 0;
}
```
2. strcpy:用于将一个字符串复制到另一个字符串
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str1[] = "Hello";
char str2[10];
strcpy(str2, str1);
cout << "The copied string is: " << str2 << endl;
return 0;
}
```
3. strcat:用于将一个字符串追加到另一个字符串的末尾
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str1[] = "Hello";
char str2[] = " world!";
strcat(str1, str2);
cout << "The concatenated string is: " << str1 << endl;
return 0;
}
```
4. toupper:用于将小写字母转换为大写字母
```c++
#include <iostream>
#include <cctype>
using namespace std;
int main() {
char ch = 'a';
char upper_ch = toupper(ch);
cout << "The uppercase letter is: " << upper_ch << endl;
return 0;
}
```
5. pow:用于计算幂次方
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
cout << "The result is: " << result << endl;
return 0;
}
```
6. abs:用于计算绝对值
```c++
#include <iostream>
#include <cstdlib>
using namespace std;
int main() {
int x = -5;
int abs_x = abs(x);
cout << "The absolute value of " << x << " is: " << abs_x << endl;
return 0;
}
```
7. atoi:用于将字符串转换为整数
```c++
#include <iostream>
#include <cstdlib>
using namespace std;
int main() {
char str[] = "123";
int num = atoi(str);
cout << "The converted integer is: " << num << endl;
return 0;
}
```
8. atof:用于将字符串转换为浮点数
```c++
#include <iostream>
#include <cstdlib>
using namespace std;
int main() {
char str[] = "3.14";
double num = atof(str);
cout << "The converted float is: " << num << endl;
return 0;
}
```
9. sqrt:用于计算平方根
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double x = 4.0;
double square_root = sqrt(x);
cout << "The square root of " << x << " is: " << square_root << endl;
return 0;
}
```
10. ceil:用于向上取整
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double x = 3.14;
double ceil_x = ceil(x);
cout << "The ceiling of " << x << " is: " << ceil_x << endl;
return 0;
}
```
11. floor:用于向下取整
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double x = 3.14;
double floor_x = floor(x);
cout << "The floor of " << x << " is: " << floor_x << endl;
return 0;
}
```
12. round:用于四舍五入
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double x = 3.6;
double round_x = round(x);
cout << "The rounded value of " << x << " is: " << round_x << endl;
return 0;
}
```
13. srand:用于初始化随机数种子
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
srand(time(0)); // 初始化随机数种子
int random_num = rand() % 100; // 生成一个0-99的随机数
cout << "The random number is: " << random_num << endl;
return 0;
}
```
14. getline:用于读取一行字符串
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
cout << "Enter a string: ";
getline(cin, str);
cout << "The string you entered is: " << str << endl;
return 0;
}
```
15. cin.ignore:用于忽略输入流中的字符
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
srand(time(0)); // 初始化随机数种子
int random_num;
cout << "Guess a number between 1 and 100: ";
cin >> random_num;
cin.ignore(100, '\n'); // 忽略输入流中的字符
int actual_num = rand() % 100 + 1;
if (random_num == actual_num) {
cout << "Congratulations! You guessed the number!" << endl;
} else {
cout << "Sorry, the actual number was: " << actual_num << endl;
}
return 0;
}
```
16. isalpha:用于判断字符是否为字母
```c++
#include <iostream>
#include <cctype>
using namespace std;
int main() {
char ch = 'a';
if (isalpha(ch)) {
cout << ch << " is a letter" << endl;
} else {
cout << ch << " is not a letter" << endl;
}
return 0;
}
```
17. isdigit:用于判断字符是否为数字
```c++
#include <iostream>
#include <cctype>
using namespace std;
int main() {
char ch = '5';
if (isdigit(ch)) {
cout << ch << " is a digit" << endl;
} else {
cout << ch << " is not a digit" << endl;
}
return 0;
}
```
18. to_string:用于将数字转换为字符串
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
int num = 123;
string str = to_string(num);
cout << "The converted string is: " << str << endl;
return 0;
}
```
19. stoi:用于将字符串转换为整数
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "123";
int num = stoi(str);
cout << "The converted integer is: " << num << endl;
return 0;
}
```
20. stof:用于将字符串转换为浮点数
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "3.14";
float num = stof(str);
cout << "The converted float is: " << num << endl;
return 0;
}
```
阅读全文
相关推荐
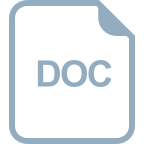
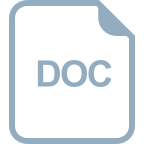

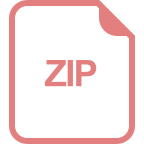
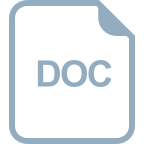
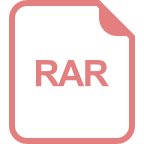
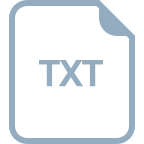
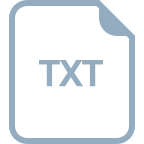
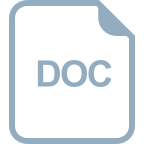
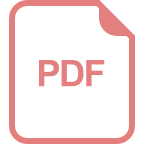
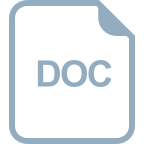
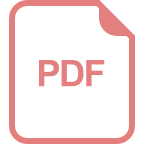
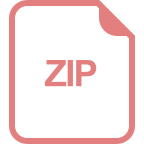
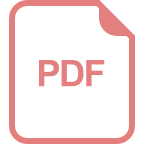
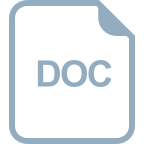
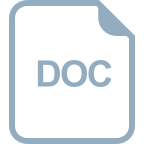
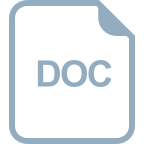
