.有三个学生,各四门课,定义二维数组score[3][4]来存放成绩。要求找出其中有不及格课程的学生并输出该学生的全部成绩。要求编写返回指针的函数search来查询学生成绩。
时间: 2024-12-05 21:15:36 浏览: 22
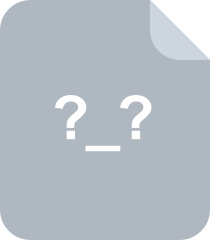
C语言程序设计-有一个一维数组score,内放10个学生的成绩,用一个函数来求平均成绩;

为了查找有不及格课程的学生及其所有成绩,我们可以定义一个`search`函数,它接受一个二维整数数组`score`作为输入,表示学生的成绩,并遍历数组来寻找不及格的课程。如果找到不及格(通常低于某个特定分数标准,比如60分),则返回指向含有该学生所有成绩信息的行指针。如果没有发现不及格课程,则返回NULL。
```c++
#include <stdio.h>
#include <stdbool.h>
// 假设及格分数为60
const int PASS_SCORE = 60;
// 结构体用于存储单个学生的信息
typedef struct {
int studentIndex; // 学生索引
int scores[4]; // 成绩数组
} Student;
// 函数返回包含不及格课程学生信息的Student*指针,如果不存在则返回NULL
Student* search(int score[][4], int studentsCount) {
for (int i = 0; i < studentsCount; ++i) {
bool hasFailed = false;
for (int j = 0; j < 4; ++j) {
if (score[i][j] < PASS_SCORE) {
hasFailed = true;
break;
}
}
if (hasFailed) {
Student* result = (Student*)malloc(sizeof(Student));
result->studentIndex = i;
result->scores = score[i];
return result;
}
}
// 没有找到不及格课程的学生,返回NULL
return NULL;
}
// 示例用法
int main() {
int score[3][4] = {
{85, 90, 75, 80},
{95, 100, 92, 98},
{65, 70, 55, 60}
};
Student* failedStudent = search(score, 3);
if (failedStudent != NULL) {
printf("Failed student index: %d\n", failedStudent->studentIndex);
for (int k = 0; k < 4; ++k) {
printf("Score: %d ", failedStudent->scores[k]);
}
free(failedStudent); // 释放内存
} else {
printf("No failed student found.\n");
}
return 0;
}
阅读全文
相关推荐
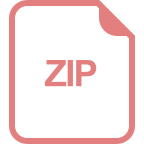
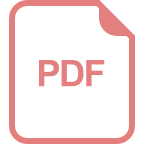














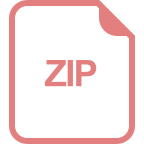
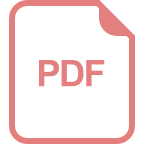