编译原理设计LL(1)文法分析器全部代码C++
时间: 2024-12-18 07:29:47 浏览: 34
编译原理中的LL(1)分析器是一种简单的自底向上的解析技术,主要用于识别左递归或无右递归的文法。这里简要描述一下构建一个基本的LL(1)文法分析器的C++代码框架,并不是完整代码,因为这需要大量的细节和库支持(如堆栈、状态机等)。核心步骤包括:
1. 定义语法符号( terminals 和 non-terminals):
```cpp
enum Symbol { ... };
```
2. 定义状态机(States)和动作表(Action Table):
```cpp
struct State {
// 状态ID
int id;
// 动作列表
std::map<Symbol, Action> actions;
};
// 动作类型
enum ActionType { Shift, Reduce, Accept };
```
3. 创建解析器函数:
```cpp
std::vector<State> states;
void build_LL1_Analyzer();
State* parse LL1(State*, const std::string&);
```
4. 构建状态转移表(state transition function):
```cpp
Action parse(State* current, Symbol symbol) {
auto it = current->actions.find(symbol);
if (it != current->actions.end()) return it->second;
// 如果找不到则处理默认情况...
}
```
5. 读取输入并驱动解析过程:
```cpp
int main() {
build_LL1_Analyzer();
State initialState;
// 从输入流中获取字符并解析
for (char c : input) {
initialState = parse(&initialState, get对应的符号(c));
if (initialState == accept_state) break; // 找到接受状态则结束
}
// 返回结果...
}
```
请注意,上述代码片段只是提供了一个大概的概念,实际的LL(1)分析器编写会更复杂,涉及到错误处理、符号处理和具体的文法规则。如果你需要完整的代码示例,建议查阅相关的教材或者在线教程。
阅读全文
相关推荐
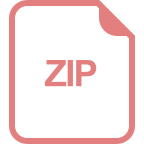
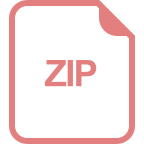
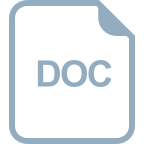
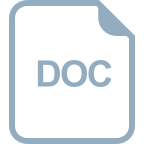
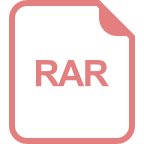
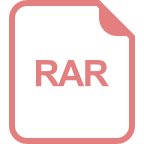
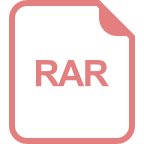
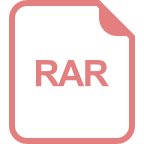
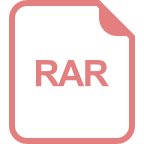
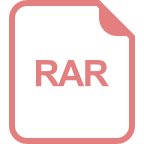
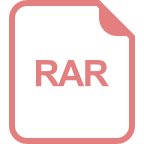
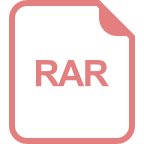
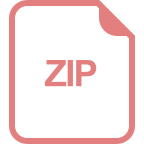
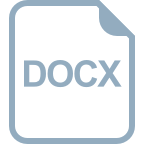
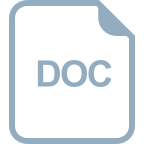
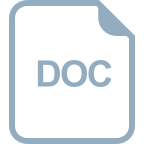
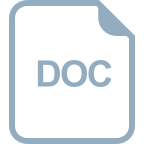