单项链表的翻转和原地翻转
时间: 2024-01-04 20:38:24 浏览: 28
对于单项链表的翻转,可以使用迭代和递归两种方法来实现。
1. 迭代方法:
迭代方法是通过使用三个指针,分别表示当前节点、当前节点的前一个节点以及当前节点的后一个节点,依次将当前节点的指针反转,直到遍历完整个链表为止。具体步骤如下:
```python
def reverse_linked_list(head):
prev = None
current = head
while current is not None:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
```
2. 递归方法:
递归方法通过不断地递归调用反转函数来实现链表的翻转。具体步骤如下:
```python
def reverse_linked_list(head):
if head is None or head.next is None:
return head
new_head = reverse_linked_list(head.next)
head.next.next = head
head.next = None
return new_head
```
以上两种方法都可以实现单项链表的翻转。另外,如果要求原地翻转链表,则迭代方法是更符合要求的,因为它只需要常数级别的额外空间。而递归方法需要使用系统栈来保存递归调用的信息,因此需要额外的空间。
相关推荐
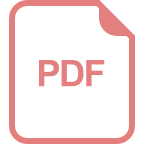
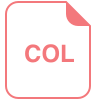
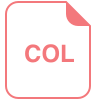
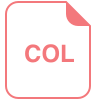
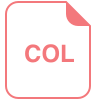
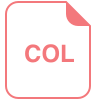







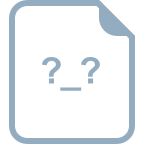