需要将/Users/gtx/Desktop/苏轼涉酒诗词.xlsx文件中的内容,涉及到/Users/gtx/Desktop/酒词语.txt中的字都用高亮标注。写出任务python代码
时间: 2024-09-25 13:05:01 浏览: 58
为了完成这个任务,我们可以使用pandas读取Excel文件,然后逐行检查诗句中是否包含文本文件中的关键词,如果包含则替换为高亮格式。这里是一个简单的Python代码示例:
```python
import pandas as pd
from termcolor import colored # 使用termcolor库进行颜色标记,需要提前安装(pip install termcolor)
# 读取关键词文件
def read_keywords_from_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
target_words = [line.strip() for line in file]
return target_words
# 高亮文本函数
def highlight_text(text, targets):
for target in targets:
text = text.replace(target, colored(target, 'red')) # 将目标词改为红色高亮
return text
# 读取Excel文件
def read_poems_from_excel(file_path, sheet_name, column_name):
df = pd.read_excel(file_path, sheet_name=sheet_name)
poems = df[column_name].tolist()
return poems
# 主程序
keywords_file_path = "/Users/gtx/Desktop/酒词语.txt"
excel_file_path = "/Users/gtx/Desktop/苏轼涉酒诗词.xlsx"
sheet_name = 'Sheet1' # 假设第一个工作表名为'Sheet1'
poem_column_name = 'Poems' # 假设包含诗句的列名为'Poems'
target_words = read_keywords_from_file(keywords_file_path)
poems = read_poems_from_excel(excel_file_path, sheet_name, poem_column_name)
highlighted_poems = []
for poem in poems:
try:
highlighted_poem = highlight_text(poem, target_words)
highlighted_poems.append(highlighted_poem)
except Exception as e:
print(f"Error processing poem: {e}")
# 打印高亮后的诗句
for i, poem in enumerate(highlighted_poems, start=1):
print(f"诗歌 {i}:")
print(poem)
```
这段代码首先定义了几个辅助函数,然后读取Excel文件和关键词文件,接着遍历Excel表格中的每一行诗句,将其与关键词对比并高亮。如果有异常发生,会打印错误信息。注意,termcolor库用于颜色标记,你需要先安装它(`pip install termcolor`),并且确保终端支持颜色输出。
阅读全文
相关推荐
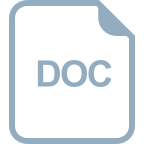
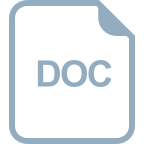
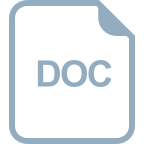
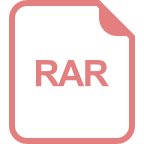
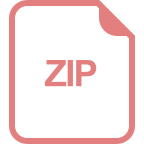
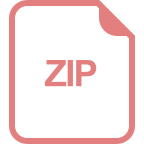
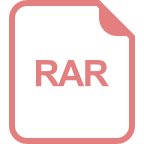
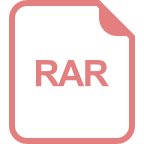
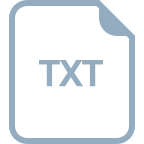
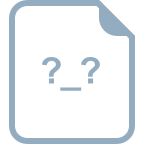
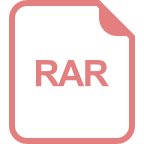
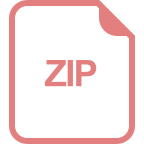
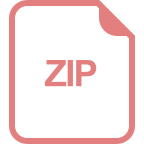
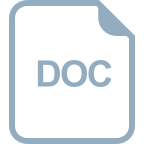
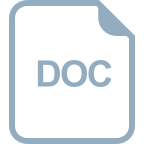