https://blog.csdn.net/myyhtw/article/details/94579126,根据这个网页,springboot netty tcp通信,创建10000个客户端,连接服务器,优化后的完整代码
时间: 2024-11-09 17:26:16 浏览: 23
这篇博客文章详细介绍了如何使用Spring Boot结合Netty框架实现实时TCP通信,并展示了如何处理大量客户端同时连接服务器的情况。优化后的Spring Boot + Netty TCP通信代码通常包括以下几个步骤:
1. 引入依赖:首先,在`pom.xml`中添加Spring Boot和Netty的相关依赖。
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
</dependency>
</dependencies>
```
2. 创建服务端处理器:建立一个处理连接的ServerHandler,处理客户端发送的消息。
```java
public class TcpServer {
private final ServerBootstrap bootstrap;
public TcpServer(int port) {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
bootstrap = new ServerBootstrap()
.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new MessageDecoder(), new MessageEncoder(), new ServerHandler());
}
});
} catch (Exception e) {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
throw e;
}
// 启动服务
bootstrap.bind(port).sync();
}
}
```
3. 客户端连接和消息传递:客户端通过Netty连接服务器并发送数据。
```java
public class TcpClient {
public static void main(String[] args) {
TcpServer server = new TcpServer(8080);
for (int i = 0; i < 10000; i++) {
createTcpClientAndConnect(server.getPort());
}
}
private static void createTcpClientAndConnect(int port) {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ClientHandler());
ChannelFuture f = b.connect(host, port).sync();
f.channel().writeAndFlush("Hello from client " + i); // 发送数据
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
}
class ClientHandler extends ChannelInboundHandlerAdapter {
//...
}
```
文章中还可能涉及到线程池管理、错误处理、消息解码/编码等细节。为了详细了解完整的代码和优化部分,建议查看原文链接提供的内容。
阅读全文
相关推荐
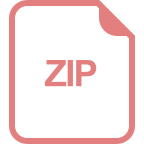
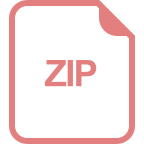
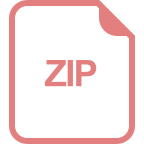

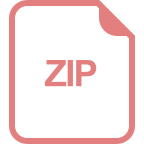
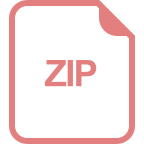
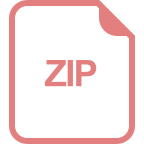







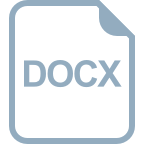