Write a program that takes a list of strings and prints the length of each string using a for loop.
时间: 2024-10-25 14:15:50 浏览: 26
To write a program in C++ that takes a list of strings and prints the length of each string using a `for` loop, you would first need to declare an array or vector of strings. Here's a simple example:
```cpp
#include <iostream>
#include <vector> // Include the library for dynamic arrays
int main() {
std::vector<std::string> stringList = {"apple", "banana", "cherry"}; // Declare a vector of strings
// Iterate through the vector using a for loop
for (const auto& str : stringList) { // Using range-based for loop for simplicity
std::cout << "Length of string \"" << str << "\": " << str.length() << std::endl;
}
return 0;
}
```
In this code snippet, we use the `std::vector` container from `<vector>` to store the strings. The `for` loop iterates over each string (`str`) in the vector, and we call the `length()` method to get the length of the string. The result is then printed to the console.
阅读全文
相关推荐
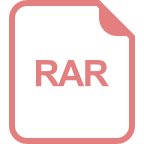
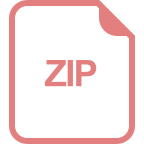

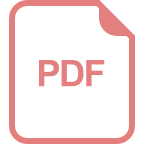
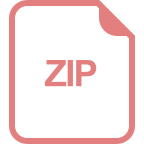
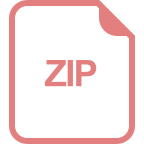
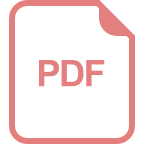
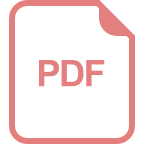
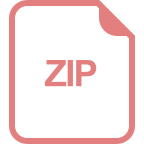
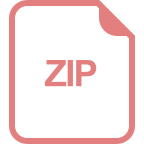
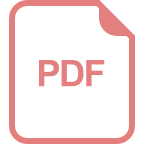
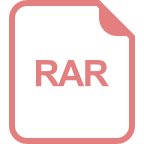
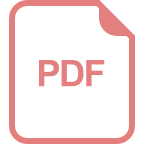
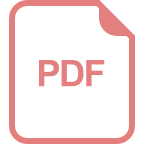
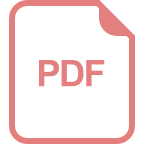