c++实现素材:1 my English teacher is 12 22 hygeagen Monika 123 3 she is a very lovely person 123 4 I love her forever;.读入一个英文文本文件,英文中有字母、数字、其它字符,无汉字,文件有多行(程序要能处理至少4行文字,附件中有参考素材)。 2.编写函数实现:统计字母的出现频度,并显示结果。(大小写算同一字母) 3.编写函数实现:统计其中单词的个数,并显示结果。(单词个数以WORD统计作为参考,不一定100%相同。) 4.编写函数实现:找出最长的单词,并显示单词及其长度。 5.编写函数实现:统计出现的整数,存入一维数组,并显示结果。 (1) 比如文件中有两行: "abc 123 W 6 www 9." "teacher 10 student 7." (2) 分析得到5个数:123、6、9、10、7。 (3) 定义数组int a[1000] (4) a[0]=123,a[1]=6,a[2]=9,a[3]=10,a[4]=7。 注:可以利用下标或字符型指针处理字符串
时间: 2024-02-03 08:11:36 浏览: 147
以下是实现上述要求的C++代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <cstring>
using namespace std;
// 统计字母的出现频度
void countLetters(string text) {
int freq[26] = {0};
for (int i = 0; i < text.length(); i++) {
if (isalpha(text[i])) {
freq[tolower(text[i]) - 'a']++;
}
}
for (int i = 0; i < 26; i++) {
if (freq[i] > 0) {
cout << char('a' + i) << " : " << freq[i] << endl;
}
}
}
// 统计单词的个数
int countWords(string text) {
int count = 0;
bool inWord = false;
for (int i = 0; i < text.length(); i++) {
if (isalpha(text[i])) {
if (!inWord) {
count++;
inWord = true;
}
}
else {
inWord = false;
}
}
return count;
}
// 找出最长的单词
string findLongestWord(string text) {
string longestWord = "";
string currentWord = "";
for (int i = 0; i < text.length(); i++) {
if (isalpha(text[i])) {
currentWord += tolower(text[i]);
}
else {
if (currentWord.length() > longestWord.length()) {
longestWord = currentWord;
}
currentWord = "";
}
}
if (currentWord.length() > longestWord.length()) {
longestWord = currentWord;
}
return longestWord;
}
// 统计出现的整数
void findIntegers(string text, int a[]) {
int count = 0;
string currentNum = "";
for (int i = 0; i < text.length(); i++) {
if (isdigit(text[i])) {
currentNum += text[i];
}
else {
if (currentNum != "") {
a[count++] = stoi(currentNum);
currentNum = "";
}
}
}
if (currentNum != "") {
a[count++] = stoi(currentNum);
}
for (int i = 0; i < count; i++) {
cout << a[i] << " ";
}
cout << endl;
}
int main() {
ifstream infile("text.txt");
string line;
int a[1000];
int count = 0;
while (getline(infile, line)) {
cout << "Text: " << line << endl;
countLetters(line);
int wordCount = countWords(line);
cout << "Word count: " << wordCount << endl;
string longestWord = findLongestWord(line);
cout << "Longest word: " << longestWord << " (" << longestWord.length() << " letters)" << endl;
findIntegers(line, a);
count += countWords(line);
}
cout << "Total word count: " << count << endl;
return 0;
}
```
解释一下代码的实现:
- 首先,我们使用`ifstream`读入文件中的文本。
- 然后,我们对于每一行文本,分别进行字母出现频度统计、单词个数统计、最长单词查找和整数统计。
- 字母出现频度统计使用一个大小为26的数组,将每个字母出现的次数累加到对应的数组元素中即可。
- 单词个数统计使用一个布尔变量`inWord`来判断当前字符是否在单词中,在单词中则不累加计数器,否则计数器加1。
- 最长单词查找使用两个字符串变量,一个存储当前单词,一个存储最长单词。遍历文本,当遇到非字母字符时,比较当前单词的长度与最长单词的长度,如果当前单词更长,则更新最长单词。
- 整数统计使用一个数组和一个计数器,遍历文本,当遇到数字字符时,将其添加到一个字符串变量`currentNum`中,当遇到非数字字符时,将`currentNum`转换成整数,存入数组,并将`currentNum`清空。遍历完毕后,输出整数数组中的所有元素。
- 最后,我们统计所有行文本的单词个数,并输出。
以上是实现要求的代码,希望能对你有所帮助!
阅读全文
相关推荐
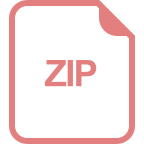
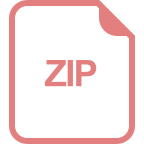
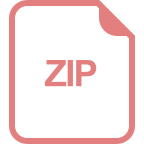
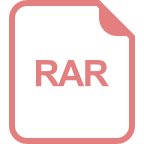
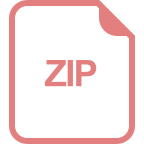
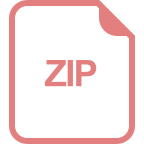
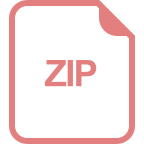
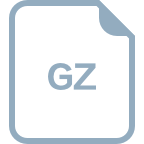
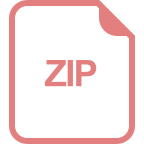
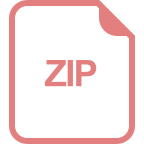
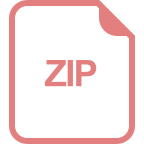
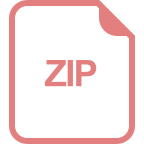
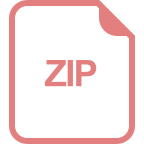
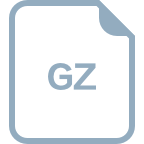
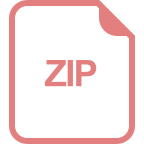
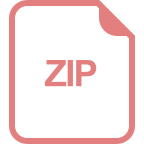
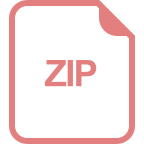