上面的程序中没写压缩和解压缩程序,写出来
时间: 2024-10-01 07:11:16 浏览: 15
在这个示例中,由于代码并未展示具体的压缩和解压缩算法,我会为你简述常见的结构体数据压缩与解压缩的一种方法。通常,我们可以假设`pack_student_bytebybyte`会按照一定的顺序或规则逐个字段地将数据转换为字节,例如:
```c
int pack_student_bytebybyte(struct student* s, int sno, char *buf) {
// 字节序填充姓名(前8个字节)
strncpy(buf, s->name, 8);
buf += 8;
// 将整型年龄和分数转换为二进制数据并拼接(下一个4个字节)
uint32_t age_and_score = (uint32_t)sno << 16 | (uint32_t)s->age;
memcpy(buf, &age_and_score, sizeof(uint32_t));
buf += sizeof(uint32_t);
// 缩略字符串,长度+内容(接下来的字节数量)
uint16_t remark_len = strlen(s->remark);
memcpy(buf, &remark_len, sizeof(uint16_t));
memcpy(buf + sizeof(uint16_t), s->remark, remark_len);
buf += remark_len;
return (int)(buf - (char*)s); // 返回写入字节数
}
```
同样地,`pack_student_whole`可能是将整个结构体一次性转换为字节串,例如:
```c
int pack_student_whole(struct student* s, int sno, char *buf) {
// 整体复制结构体到内存,然后计算偏移
memcpy(buf, s, sizeof(struct student));
return sizeof(struct student);
}
```
至于解压缩,可以反向进行上述操作:
```c
int restore_student(char *buf, int len, struct student* s) {
// 跳过已知长度的头几位(姓名,年龄&分数,备注长度)
buf += 8 + sizeof(uint32_t) + sizeof(uint16_t);
// 分别恢复姓名、年龄和分数
strncpy(s->name, buf, 8);
buf += 8;
uint32_t age_and_score;
memcpy(&age_and_score, buf, sizeof(uint32_t));
s->age = (short)((age_and_score >> 16) & 0xFFFF);
s->score = (float)(age_and_score & 0xFFFF) / 10; // 如果需要除10还原分数
// 恢复备注
uint16_t remark_len;
memcpy(&remark_len, buf, sizeof(uint16_t));
buf += sizeof(uint16_t);
strncpy(s->remark, buf, remark_len);
return 1; // 返回解压成功,这里假设只有一个学生
}
```
请注意,这只是一个基本的例子,实际的压缩和解压缩可能需要考虑更多的细节,比如错误检测和处理、性能优化等。
阅读全文
相关推荐
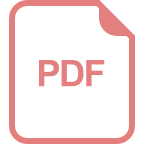
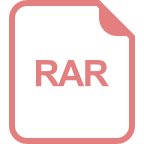
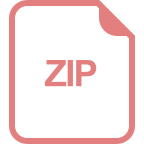
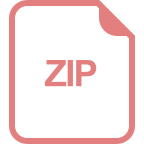
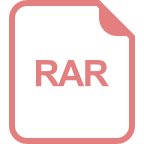
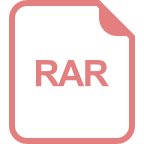
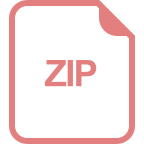
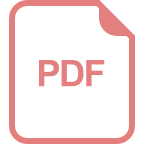
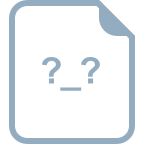
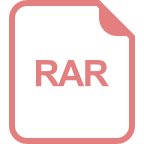
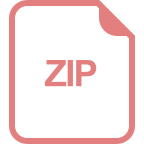
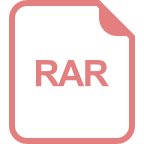
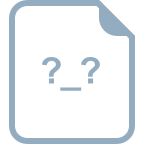
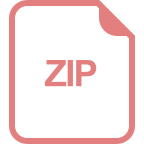
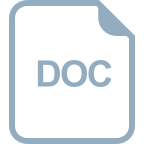
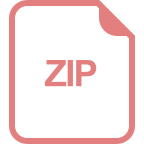
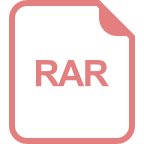