如何根据人的身高体重计算BMI值并生成一个平面的仿真人体用python的第三方库
时间: 2024-03-10 09:44:12 浏览: 131
可以使用Python的第三方库PyQt5来生成一个平面的仿真人体。PyQt5是一个流行的Python GUI工具包,它提供了丰富的图形界面组件和工具,可以用于创建各种GUI应用程序,包括2D和3D图形。
以下是Python代码示例,可以根据输入的身高体重计算BMI值并生成一个平面的仿真人体:
```python
import sys
from PyQt5.QtWidgets import QApplication, QGraphicsScene, QGraphicsView, QGraphicsEllipseItem, \
QGraphicsRectItem, QGraphicsTextItem, QGraphicsItemGroup
from PyQt5.QtGui import QBrush, QColor, QFont
class BodyView(QGraphicsView):
def __init__(self, parent=None):
super().__init__(parent)
self.scene = QGraphicsScene(self)
self.setScene(self.scene)
self.draw_body()
def draw_body(self):
# 输入身高和体重
height = float(input("请输入身高(米):"))
weight = float(input("请输入体重(千克):"))
# 计算BMI值
bmi = weight / (height ** 2)
# 计算人体各部位的尺寸
head_width = 0.3 * height
head_height = 0.2 * height
neck_width = 0.2 * height
torso_width = 0.4 * height
torso_height = 0.6 * height
arm_width = 0.15 * height
arm_height = 0.5 * height
leg_width = 0.2 * height
leg_height = 0.6 * height
# 创建各部位的图形项
head = QGraphicsEllipseItem(-head_width / 2, -head_height / 2, head_width, head_height)
neck = QGraphicsRectItem(-neck_width / 2, head_height / 2, neck_width, head_height / 4)
torso = QGraphicsRectItem(-torso_width / 2, head_height * 3 / 4, torso_width, torso_height)
left_arm = QGraphicsRectItem(-torso_width / 2 - arm_width, head_height * 3 / 4 + arm_height / 4, arm_width, arm_height)
right_arm = QGraphicsRectItem(torso_width / 2, head_height * 3 / 4 + arm_height / 4, arm_width, arm_height)
left_leg = QGraphicsRectItem(-leg_width / 2, head_height * 3 / 4 + torso_height, leg_width, leg_height)
right_leg = QGraphicsRectItem(leg_width / 2, head_height * 3 / 4 + torso_height, leg_width, leg_height)
# 将各部位的图形项组合为一个整体
body = QGraphicsItemGroup()
body.addToGroup(head)
body.addToGroup(neck)
body.addToGroup(torso)
body.addToGroup(left_arm)
body.addToGroup(right_arm)
body.addToGroup(left_leg)
body.addToGroup(right_leg)
# 根据BMI值填充颜色
if bmi < 18.5:
brush = QBrush(QColor("blue"))
elif bmi < 24:
brush = QBrush(QColor("green"))
elif bmi < 28:
brush = QBrush(QColor("orange"))
else:
brush = QBrush(QColor("red"))
body.setBrush(brush)
# 设置整体位置和大小
body.setPos(self.width() / 2, self.height() / 2)
body.setScale(2)
# 显示BMI值
text = QGraphicsTextItem("BMI = {:.2f}".format(bmi))
text.setFont(QFont("Arial", 16))
text.setPos(self.width() / 2, self.height() / 2 + head_height / 2 + 20)
self.scene.addItem(text)
if __name__ == '__main__':
app = QApplication(sys.argv)
view = BodyView()
view.show()
sys.exit(app.exec_())
```
运行代码后,会让用户输入身高和体重,然后根据计算出的BMI值来填充相应的颜色,最后显示BMI值。在PyQt5中,我们首先创建了一个QGraphicsView对象,并在其中创建一个QGraphicsScene对象。然后,我们使用QGraphicsEllipseItem、QGraphicsRectItem和QGraphicsTextItem等图形项来创建各部位的图形,并将它们组合为一个整体。最后,我们将整体添加到QGraphicsScene对象中,并在QGraphicsView对象中显示它。
阅读全文
相关推荐
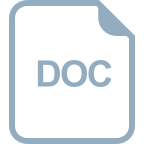
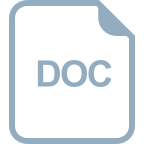
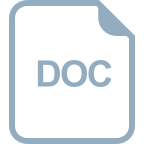















