将SWT的Table的数据存入sql中
时间: 2023-11-27 13:49:40 浏览: 35
要将 SWT 的 Table 的数据存入 SQL 中,可以按照以下步骤进行操作:
1. 首先,获取 SWT 的 Table 中的数据。可以通过 Table#getItems() 方法获取 Table 中的所有行。
2. 创建 SQL 的连接。可以使用 JDBC 来连接 SQL 数据库。具体连接方法可以参考 JDBC 的文档和示例。
3. 使用 SQL 的 INSERT 语句将数据插入到数据库中。可以使用 PreparedStatement 来执行 INSERT 语句,并将 Table 中的每一行数据插入到数据库中。
下面是一个简单的示例代码,演示如何将 SWT 的 Table 中的数据存入 SQL 中:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Table;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.swt.widgets.TableItem;
public class TableToSqlExample {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setLayout(new FillLayout());
// 创建 Table,并添加表头
Table table = new Table(shell, SWT.BORDER | SWT.FULL_SELECTION);
table.setHeaderVisible(true);
table.setLinesVisible(true);
TableColumn column1 = new TableColumn(table, SWT.NONE);
TableColumn column2 = new TableColumn(table, SWT.NONE);
column1.setText("Name");
column2.setText("Age");
column1.setWidth(100);
column2.setWidth(100);
// 添加数据
TableItem item1 = new TableItem(table, SWT.NONE);
item1.setText(new String[] { "John", "25" });
TableItem item2 = new TableItem(table, SWT.NONE);
item2.setText(new String[] { "Mary", "30" });
// 将数据存入 SQL
try {
// 创建 SQL 连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
// 创建 PreparedStatement
String sql = "INSERT INTO person (name, age) VALUES (?, ?)";
PreparedStatement stmt = conn.prepareStatement(sql);
// 遍历 Table 中的每一行,将数据插入到数据库中
for (TableItem item : table.getItems()) {
String name = item.getText(0);
int age = Integer.parseInt(item.getText(1));
stmt.setString(1, name);
stmt.setInt(2, age);
stmt.executeUpdate();
}
// 关闭连接和语句
stmt.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
shell.pack();
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
```
其中,假设数据库中已经有一个名为 `test` 的数据库,其中有一个名为 `person` 的表,该表有两个字段 `name` 和 `age`,分别表示人名和年龄。在上述示例代码中,我们遍历了 Table 中的每一行,将人名和年龄插入到 `person` 表中。注意,该示例代码仅作为演示用途,实际使用时需根据具体情况进行修改。
相关推荐
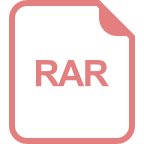
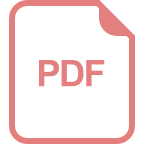














