下列选项中,能够读取内部存储文件中数据的类是()。 A.FilelnputStream B.InputStream C.FileOutputStream D.OutputStream
时间: 2023-08-25 12:28:37 浏览: 88
正确的选项是A。`FileInputStream` 类是 Java 中用于读取文件数据的类之一,可以用于读取 Android 应用程序的内部存储中的文件数据。而 `InputStream` 和 `OutputStream` 类是抽象类,不能直接用于文件读写操作;`FileOutputStream` 类则是用于写入文件数据的类。因此,选项A描述正确,而B、C、D都是错误的。
相关问题
java inputstream子类_Java流操作,InputStream、OutputStream及子类FileInputStream、FileOutputStream;BufferedInpu...
Java中的流(stream)是一种用于处理输入输出操作的抽象数据类型。InputStream和OutputStream是Java中最基本的流,它们分别用于读取和写入字节流。FileInputStream和FileOutputStream是InputStream和OutputStream的子类,用于读取和写入文件。BufferedInputStream和BufferedOutputStream是对InputStream和OutputStream的包装,用于提高读写效率。下面我们来简单介绍一下这些类的用法。
1. InputStream:用于读取字节流,包含多个抽象方法,如read()、available()等。
2. OutputStream:用于写入字节流,包含多个抽象方法,如write()、flush()等。
3. FileInputStream:用于读取文件,继承自InputStream,可以直接读取文件中的字节数据。
4. FileOutputStream:用于写入文件,继承自OutputStream,可以直接将字节数据写入文件。
5. BufferedInputStream:用于提高读取效率,包装了InputStream,提供了缓冲区功能。
6. BufferedOutputStream:用于提高写入效率,包装了OutputStream,提供了缓冲区功能。
使用这些类时,需要注意关闭流以释放资源。可以使用try-with-resources语句来自动关闭流,也可以在finally块中手动关闭流。示例代码如下:
```
try(InputStream input = new FileInputStream("file.txt");
OutputStream output = new FileOutputStream("output.txt")) {
// 读取文件并写入到输出流
} catch(IOException e) {
// 处理异常
} finally {
// 关闭流
}
```
android自定义rc文件,如何使用android init.rc(vendor.rc)读取文件中的值
在 Android 系统中,init.rc 是 Android 系统启动时的初始化脚本,它负责启动 Android 系统中的各个服务和进程,并设置系统的一些属性。
如果你想在 init.rc 或 vendor.rc 中读取自定义的 rc 文件中的值,可以通过以下步骤实现:
1. 在 Android 应用程序的 assets 文件夹中创建一个自定义的 rc 文件,比如 myrc.rc,将需要设置的属性值写入该文件中。
2. 在 Android 应用程序的代码中,使用 AssetManager 类读取该文件:
```java
AssetManager assetManager = context.getAssets();
InputStream inputStream = assetManager.open("myrc.rc");
```
3. 将 inputStream 中的数据读取到一个字符串中:
```java
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder builder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
builder.append(line);
}
String rcContent = builder.toString();
```
4. 将读取到的字符串写入到 /data/local/tmp/myrc.rc 文件中:
```java
File file = new File("/data/local/tmp/myrc.rc");
FileOutputStream outputStream = null;
try {
outputStream = new FileOutputStream(file);
outputStream.write(rcContent.getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
if (outputStream != null) {
try {
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
5. 在 init.rc 或 vendor.rc 中使用 source 命令读取该文件中的属性值:
```
source /data/local/tmp/myrc.rc
```
6. 在 Android 系统启动时,init.rc 或 vendor.rc 会执行 source 命令,读取 /data/local/tmp/myrc.rc 文件中的属性值并设置系统属性。
注意,由于 /data/local/tmp 目录是临时目录,系统重启后该文件会被清除,因此每次系统启动时都需要重新执行以上步骤来读取自定义的 rc 文件中的属性值。
相关推荐
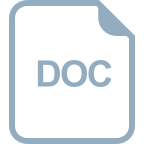
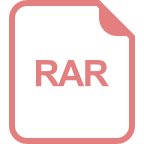
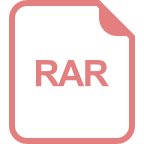












