c++中,需要实现如下功能:数据源每一帧会给予一个类,这个类有多个成员变量,我们要把这个类里的每个成员变量的值保存成数组的形式,而且成员变量名也要保存下来
时间: 2024-03-31 12:33:56 浏览: 14
您可以通过使用反射来实现这个功能。在 C++ 中,反射可以使用模板元编程来实现。您可以使用类模板和成员变量模板来让程序在编译期间自动地将类的成员变量名和值保存到数组中。
下面是一个简单的例子:
```c++
#include <iostream>
#include <array>
#include <string>
template <class T>
struct Reflection {
static constexpr size_t size = sizeof(T) / sizeof(int);
static std::array<int, size> values;
static std::array<std::string, size> names;
};
template <class T>
std::array<int, Reflection<T>::size> Reflection<T>::values{};
template <class T>
std::array<std::string, Reflection<T>::size> Reflection<T>::names{};
struct MyStruct {
int a = 1;
float b = 2.0f;
double c = 3.0;
char d = 'd';
};
template <>
struct Reflection<MyStruct> {
static constexpr size_t size = sizeof(MyStruct) / sizeof(int);
static std::array<int, size> values;
static std::array<std::string, size> names;
};
template <>
std::array<int, Reflection<MyStruct>::size> Reflection<MyStruct>::values{};
template <>
std::array<std::string, Reflection<MyStruct>::size> Reflection<MyStruct>::names{ "a", "b", "c", "d" };
template <>
struct Reflection<int> {
static constexpr size_t size = 1;
static std::array<int, size> values;
static std::array<std::string, size> names;
};
template <>
std::array<int, Reflection<int>::size> Reflection<int>::values{ 42 };
template <>
std::array<std::string, Reflection<int>::size> Reflection<int>::names{ "the answer" };
int main() {
MyStruct s;
Reflection<MyStruct>::values = { s.a, static_cast<int>(s.b), static_cast<int>(s.c), s.d };
for (size_t i = 0; i < Reflection<MyStruct>::size; ++i) {
std::cout << Reflection<MyStruct>::names[i] << ": " << Reflection<MyStruct>::values[i] << std::endl;
}
std::cout << Reflection<int>::names[0] << ": " << Reflection<int>::values[0] << std::endl;
return 0;
}
```
在这个例子中,我们定义了一个名为 `MyStruct` 的结构体,它有四个成员变量。我们还定义了一个名为 `Reflection` 的类模板,它有两个静态成员变量 `values` 和 `names`,它们分别保存了类的成员变量的值和名称。我们使用了一个特化版本的 `Reflection` 模板来处理 `MyStruct` 类型和 `int` 类型。
在 `main` 函数中,我们创建了一个 `MyStruct` 类型的对象 `s`,并将它的成员变量的值保存到 `Reflection<MyStruct>::values` 数组中。然后我们遍历这个数组并输出每个成员变量的名称和值。
这个例子只是一个简单的实现,您可以根据自己的需求来修改代码。
相关推荐
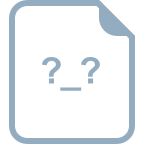
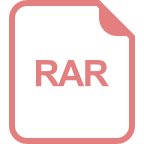
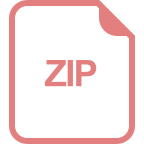














