springboot 静态方法调用service
时间: 2023-10-14 13:17:00 浏览: 318
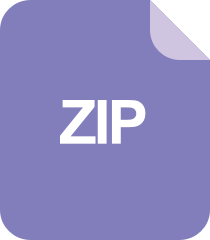
基于springboot的车牌识别系统源码.zip
在 Spring Boot 中,我们通常使用依赖注入的方式来调用 Service 类的方法,而不是直接调用静态方法。这是因为依赖注入可以更好地管理类之间的依赖关系,使得代码更易于测试和维护。
如果你非常需要调用 Service 类的静态方法,可以考虑将 Service 类的方法声明为静态方法,并在需要调用的地方直接使用类名来调用。请注意,这种做法可能会增加代码的耦合性和难以测试性。
以下是一个简单的示例,演示如何在 Spring Boot 中调用 Service 类的静态方法:
```java
@Service
public class MyService {
public static void myStaticMethod() {
// 静态方法的实现代码
}
}
@RestController
public class MyController {
@GetMapping("/test")
public String test() {
MyService.myStaticMethod(); // 直接调用静态方法
return "success";
}
}
```
请注意,虽然这种方式可以在代码中直接调用静态方法,但这并不是最优雅的解决方案。在实际开发中,我们应该尽量避免直接调用静态方法,而考虑采用依赖注入的方式来管理类之间的依赖关系。
阅读全文
相关推荐
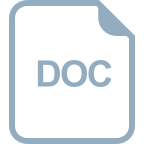
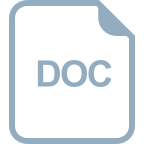
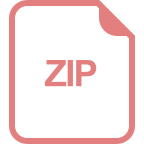
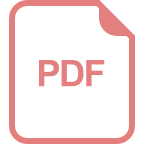
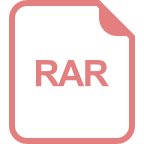
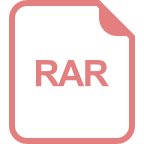
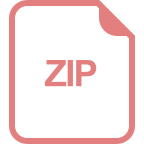
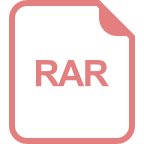
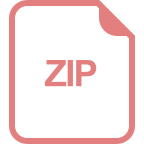
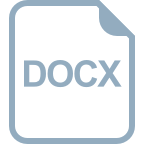
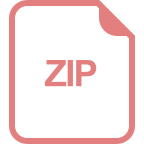
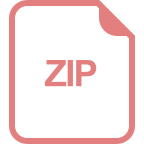





