使用Android studio编写点击按钮打开相机程序的代码要求用到registerForActivityResult 并写出操作步骤
时间: 2024-05-01 12:20:21 浏览: 13
在使用 `registerForActivityResult` 来启动相机应用程序时,需要执行以下操作步骤:
1. 在 `onCreate` 方法中初始化相机请求的 `ActivityResultLauncher` 对象,例如:
```java
// 定义一个 ActivityResultLauncher 对象
ActivityResultLauncher<Uri> cameraLauncher = registerForActivityResult(new ActivityResultContracts.TakePicture(), result -> {
if (result) {
// 处理相机拍摄的图片
}
});
```
2. 在按钮的点击事件中调用 `cameraLauncher.launch` 方法来启动相机应用程序:
```java
// 启动相机应用程序
cameraLauncher.launch(photoUri);
```
其中,`photoUri` 是存储拍摄照片的 Uri 对象,可以使用 `FileProvider` 来生成。
完整的代码示例:
```java
public class MainActivity extends AppCompatActivity {
private static final String PHOTO_FILE_NAME = "photo.jpg";
private Uri photoUri;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化相机请求的 ActivityResultLauncher 对象
ActivityResultLauncher<Uri> cameraLauncher = registerForActivityResult(new ActivityResultContracts.TakePicture(), result -> {
if (result) {
// 处理相机拍摄的图片
ImageView imageView = findViewById(R.id.imageView);
imageView.setImageURI(photoUri);
}
});
// 初始化存储拍摄照片的 Uri 对象
File photoFile = new File(getExternalFilesDir(Environment.DIRECTORY_PICTURES), PHOTO_FILE_NAME);
photoUri = FileProvider.getUriForFile(this, getPackageName() + ".provider", photoFile);
// 设置按钮的点击事件
Button button = findViewById(R.id.button);
button.setOnClickListener(v -> {
// 启动相机应用程序
cameraLauncher.launch(photoUri);
});
}
}
```
相关推荐
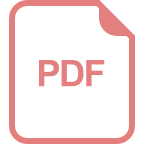
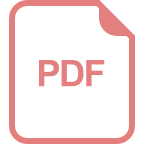














