C语言:在磁盘上创建一个 test.txt 文件,使用记事本打开后先写入 40 道单选题和相应的选项和正 确答案。再编写一个单项选择题标准化考试系统,该系统功能要求: A、 用文件保存试题库。(每个试题包括题干、4 个备选答案、标准答案)。 B、 试题录入、修改和删除。 C、 试题抽取:每次从试题库中可以随机抽出 N 道题(N 由键盘输入)。 D、 考生答题:用户抽取 N 道试题进行答题,键盘输入每道题的答案。 E、 自动判卷:系统可根据用户答案与标准答案的对比实现判卷并给出成绩。 系统操作过程中能够正确读取和更新试题文件,并进行正常的试题管理、抽取、答题和判卷 操作,该过程在控制台中显示,人机交互方便。
时间: 2024-02-06 19:10:36 浏览: 23
以下是C语言实现该考试系统的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_QUESTIONS 100
#define MAX_OPTIONS 4
#define MAX_ANSWER_LEN 2
typedef struct {
char question[100];
char options[MAX_OPTIONS][50];
char answer[MAX_ANSWER_LEN];
} Question;
void init(Question *questions, int *num_questions);
void display_menu();
void add_question(Question *questions, int *num_questions);
void edit_question(Question *questions, int num_questions);
void delete_question(Question *questions, int *num_questions);
void select_questions(Question *questions, int num_questions);
void take_exam(Question *questions, int num_questions);
int main() {
Question questions[MAX_QUESTIONS];
int num_questions = 0;
char choice;
init(questions, &num_questions);
do {
display_menu();
scanf(" %c", &choice);
switch (choice) {
case '1':
add_question(questions, &num_questions);
break;
case '2':
edit_question(questions, num_questions);
break;
case '3':
delete_question(questions, &num_questions);
break;
case '4':
select_questions(questions, num_questions);
break;
case '5':
take_exam(questions, num_questions);
break;
case '6':
printf("Goodbye!\n");
break;
default:
printf("Invalid choice. Please try again.\n");
}
} while (choice != '6');
return 0;
}
void init(Question *questions, int *num_questions) {
FILE *fp = fopen("test.txt", "r");
if (fp == NULL) {
printf("Error opening file.\n");
return;
}
char line[200];
int i = 0;
while (fgets(line, sizeof(line), fp) != NULL) {
char *token = strtok(line, ",");
strcpy(questions[i].question, token);
for (int j = 0; j < MAX_OPTIONS; j++) {
token = strtok(NULL, ",");
strcpy(questions[i].options[j], token);
}
token = strtok(NULL, ",");
strcpy(questions[i].answer, token);
(*num_questions)++;
i++;
}
fclose(fp);
}
void display_menu() {
printf("\n1. Add question\n");
printf("2. Edit question\n");
printf("3. Delete question\n");
printf("4. Select questions\n");
printf("5. Take exam\n");
printf("6. Quit\n");
printf("Enter your choice: ");
}
void add_question(Question *questions, int *num_questions) {
if (*num_questions >= MAX_QUESTIONS) {
printf("Maximum number of questions reached.\n");
return;
}
printf("Enter question: ");
getchar();
fgets(questions[*num_questions].question, sizeof(questions[*num_questions].question), stdin);
for (int i = 0; i < MAX_OPTIONS; i++) {
printf("Enter option %d: ", i + 1);
fgets(questions[*num_questions].options[i], sizeof(questions[*num_questions].options[i]), stdin);
}
printf("Enter answer: ");
scanf(" %s", questions[*num_questions].answer);
(*num_questions)++;
}
void edit_question(Question *questions, int num_questions) {
int question_num;
printf("Enter question number to edit: ");
scanf("%d", &question_num);
if (question_num < 1 || question_num > num_questions) {
printf("Invalid question number.\n");
return;
}
printf("\nQuestion: %s", questions[question_num - 1].question);
for (int i = 0; i < MAX_OPTIONS; i++) {
printf("Option %d: %s", i + 1, questions[question_num - 1].options[i]);
}
printf("Answer: %s\n", questions[question_num - 1].answer);
printf("Enter new question: ");
getchar();
fgets(questions[question_num - 1].question, sizeof(questions[question_num - 1].question), stdin);
for (int i = 0; i < MAX_OPTIONS; i++) {
printf("Enter new option %d: ", i + 1);
fgets(questions[question_num - 1].options[i], sizeof(questions[question_num - 1].options[i]), stdin);
}
printf("Enter new answer: ");
scanf(" %s", questions[question_num - 1].answer);
}
void delete_question(Question *questions, int *num_questions) {
int question_num;
printf("Enter question number to delete: ");
scanf("%d", &question_num);
if (question_num < 1 || question_num > *num_questions) {
printf("Invalid question number.\n");
return;
}
for (int i = question_num - 1; i < *num_questions - 1; i++) {
strcpy(questions[i].question, questions[i + 1].question);
for (int j = 0; j < MAX_OPTIONS; j++) {
strcpy(questions[i].options[j], questions[i + 1].options[j]);
}
strcpy(questions[i].answer, questions[i + 1].answer);
}
(*num_questions)--;
}
void select_questions(Question *questions, int num_questions) {
int num_selected;
printf("Enter number of questions to select: ");
scanf("%d", &num_selected);
if (num_selected > num_questions) {
printf("Not enough questions in database.\n");
return;
}
srand(time(NULL));
for (int i = 0; i < num_selected; i++) {
int rand_num = rand() % num_questions;
printf("\n%d. %s\n", i + 1, questions[rand_num].question);
for (int j = 0; j < MAX_OPTIONS; j++) {
printf("%c. %s", 'A' + j, questions[rand_num].options[j]);
}
}
}
void take_exam(Question *questions, int num_questions) {
int num_selected, score = 0;
printf("Enter number of questions to answer: ");
scanf("%d", &num_selected);
if (num_selected > num_questions) {
printf("Not enough questions in database.\n");
return;
}
srand(time(NULL));
for (int i = 0; i < num_selected; i++) {
int rand_num = rand() % num_questions;
char answer[MAX_ANSWER_LEN];
printf("\n%d. %s\n", i + 1, questions[rand_num].question);
for (int j = 0; j < MAX_OPTIONS; j++) {
printf("%c. %s", 'A' + j, questions[rand_num].options[j]);
}
printf("Enter your answer: ");
scanf(" %s", answer);
if (strcmp(answer, questions[rand_num].answer) == 0) {
score++;
}
}
printf("\nYou scored %d out of %d.\n", score, num_selected);
}
```
其中,`Question` 结构体表示一个试题,包括题干、备选答案和标准答案。`init` 函数从文件中读取试题库,并将每个试题保存在 `questions` 数组中,同时更新试题数量 `num_questions`。`display_menu` 函数显示菜单,让用户选择操作。`add_question` 函数让用户输入一个新的试题,并将其添加到 `questions` 数组中。`edit_question` 函数让用户选择要编辑的试题,并允许用户修改其内容。`delete_question` 函数让用户选择要删除的试题,并将其从 `questions` 数组中移除。`select_questions` 函数让用户输入要随机抽取的试题数量,并从 `questions` 数组中随机选择相应数量的试题进行展示。`take_exam` 函数让用户输入要回答的试题数量,并从 `questions` 数组中随机选择相应数量的试题进行考试,同时计算分数。
相关推荐
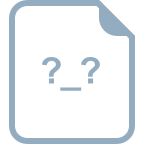















