django在数据库中根据sex字段生成不同Month下的复购率
时间: 2023-06-24 08:06:28 浏览: 49
假设你有一个名为`Order`的模型,包含`sex`和`created_time`字段,`sex`字段表示性别,`created_time`字段表示订单创建时间。你可以按照以下步骤来生成不同性别下各个月份的复购率:
1. 使用`annotate()`方法和`Count()`方法对每个月份的订单数量进行计数,并将结果保存在一个名为`order_count`的字段中:
```python
from django.db.models import Count
orders = Order.objects.annotate(order_count=Count('id', distinct=True)).all()
```
2. 将结果按照性别和月份进行分组,使用`TruncMonth()`方法将订单创建时间截断到月份级别:
```python
from django.db.models.functions import TruncMonth
grouped_orders = orders.values('sex', TruncMonth('created_time')).annotate(order_count=Count('id', distinct=True)).order_by('sex')
```
3. 对于每个性别,计算每个月份的复购率。我们可以定义一个名为`calculate_repurchase_rate()`的函数来计算复购率:
```python
import datetime
def calculate_repurchase_rate(sex, month, order_count):
current_month_orders = Order.objects.filter(sex=sex, created_time__month=month.month, created_time__year=month.year).distinct('user_id')
current_month_order_count = current_month_orders.count()
if current_month_order_count == 0:
return 0
repurchase_orders = Order.objects.filter(sex=sex, created_time__lt=month, user_id__in=current_month_orders.values_list('user_id', flat=True)).distinct('user_id')
repurchase_order_count = repurchase_orders.count()
return repurchase_order_count / current_month_order_count
```
该函数接受三个参数:性别、月份和订单数量。它首先查询给定性别和月份内的所有订单,并计算该月份内不同用户的订单数量。然后,它查询在该月份之前的所有订单,并计算哪些用户在当月再次下单。最后,它计算复购率并返回结果。
4. 对于每个分组,使用`calculate_repurchase_rate()`函数计算复购率,并将结果保存在一个名为`repurchase_rate`的字段中:
```python
result = []
for group in grouped_orders:
sex = group['sex']
month = group['created_time']
order_count = group['order_count']
repurchase_rate = calculate_repurchase_rate(sex, month, order_count)
result.append({
'sex': sex,
'month': month,
'repurchase_rate': repurchase_rate
})
```
最终,`result`列表将包含每个性别和每个月份的复购率。
相关推荐
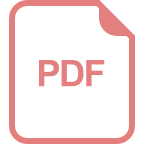
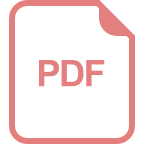














