基于VHDL的串口收发状态机实现
时间: 2023-06-15 15:05:29 浏览: 65
首先,我们需要定义串口的波特率、数据位、停止位和校验位等参数。然后,我们可以使用状态机来实现串口的收发功能。
以下是一个基于VHDL的串口状态机的实现:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
entity uart_rx is
port (
clk: in std_logic;
reset: in std_logic;
rx: in std_logic;
data: out std_logic_vector(7 downto 0);
valid: out std_logic
);
end entity;
architecture rtl of uart_rx is
-- 定义状态机的状态
type state_type is (IDLE, START, BITS, STOP, PARITY, VALID);
signal state: state_type;
-- 定义计数器和寄存器
signal cnt: integer range 0 to 15 := 0;
signal reg: std_logic_vector(7 downto 0);
begin
-- 状态机的行为
process (clk, reset)
begin
if reset = '1' then
state <= IDLE;
cnt <= 0;
reg <= (others => '0');
valid <= '0';
elsif rising_edge(clk) then
case state is
when IDLE =>
if rx = '0' then
state <= START;
cnt <= 0;
reg <= (others => '0');
end if;
when START =>
if cnt = 0 then
if rx = '0' then
cnt <= cnt + 1;
else
state <= IDLE;
end if;
else
state <= BITS;
end if;
when BITS =>
if cnt < 8 then
reg(cnt) <= rx;
cnt <= cnt + 1;
else
state <= PARITY;
end if;
when PARITY =>
if cnt = 8 then
cnt <= cnt + 1;
else
if cnt = 9 then
state <= STOP;
else
if rx = '0' then
reg(cnt-1) <= '1';
else
reg(cnt-1) <= '0';
end if;
cnt <= cnt + 1;
end if;
end if;
when STOP =>
if cnt = 10 then
state <= VALID;
else
state <= IDLE;
end if;
when VALID =>
data <= reg;
valid <= '1';
state <= IDLE;
end case;
end if;
end process;
end architecture;
```
该状态机包含以下状态:
- IDLE:空闲状态,等待接收到起始位。
- START:接收到起始位后,开始接收数据位。
- BITS:接收数据位。
- PARITY:接收校验位。
- STOP:接收停止位。
- VALID:接收到完整的数据帧,输出数据和有效信号。
该状态机使用计数器和寄存器来存储接收到的数据。当接收到完整的数据帧时,将数据和有效信号输出。在接收过程中,如果接收到错误的数据,则会重新回到空闲状态等待下一帧的接收。
需要注意的是,该状态机仅实现了串口的接收功能。如果需要实现串口的发送功能,可以采用类似的状态机设计,并在相应的状态下输出发送的数据。
相关推荐
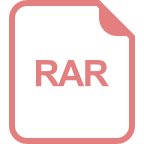
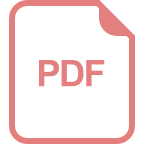














