title = soup.find("title").text
时间: 2023-07-21 20:36:52 浏览: 45
这行代码使用了BeautifulSoup库的find()方法来查找HTML页面中的title标签,并使用text属性获取其文本内容。如果该代码行出现错误,建议检查以下几点:
1. 确认你已经正确地导入了BeautifulSoup库,并使用了正确的解析器。例如,如果你的HTML页面是使用lxml解析器生成的,则应该使用BeautifulSoup(page_content, 'lxml')来初始化BeautifulSoup对象。
2. 确认HTML页面中确实包含title标签。如果没有title标签,那么find()方法将返回None,而调用text属性将会导致 'NoneType' object has no attribute 'text' 错误。
3. 确认你正在处理的HTML页面的编码与你的代码中使用的编码方式一致。这可以通过在BeautifulSoup初始化时指定编码方式来解决,例如:BeautifulSoup(page_content, 'html.parser', from_encoding='utf-8')。
如果你能够提供更多上下文或错误信息,我可以提供更具体的建议。
相关问题
title = soup.title.string 报错了
这个错误可能是因为soup没有找到title标签,或者title标签内没有文本内容。你可以通过print(soup)来查看soup的内容,确认是否有title标签存在。如果确实存在,你可以尝试使用soup.title.text或soup.find("title").text来获取标签内的文本内容。如果还是不行,可能需要检查BeautifulSoup库是否正确安装。
title = soup.find('span', class_='title').text.strip()报错'NoneType' object has no attribute 'text'
这个错误通常是因为 `soup.find()` 没有找到指定的元素,返回了 `None` 值。你可以在使用 `text` 属性之前,先判断一下返回的元素是否为 `None`,例如:
```python
title = soup.find('span', class_='title')
if title is not None:
title = title.text.strip()
else:
# 处理找不到元素的情况
```
如果你确定这个元素一定存在,可以使用 `find()` 方法的另一个版本 `find_all()`,它会返回符合条件的所有元素,你可以取第一个元素来避免这个错误:
```python
title = soup.find_all('span', class_='title')[0].text.strip()
```
相关推荐
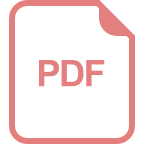
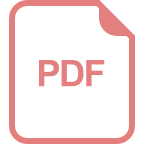
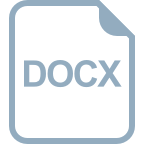












