windows下python与c++之间使用共享内存通信,python为服务器,c++为客户端可以进行信息交互。代码实现
时间: 2023-07-14 15:12:43 浏览: 217
下面是一个简单的示例代码,其中Python作为服务器,C++作为客户端,使用共享内存进行通信:
Python服务器端代码:
```python
import mmap
import os
# 创建一个共享内存区
shm = mmap.mmap(-1, 1024, "PythonServer")
shm.seek(0)
while True:
# 读取客户端发送的数据
data = shm.readline().strip()
if not data:
break
print("Received data:", data)
# 将处理后的数据发送给客户端
response = "Hello, " + data.decode()
shm.seek(0)
shm.write(response.encode())
# 关闭共享内存区
shm.close()
```
C++客户端代码:
```c++
#include <iostream>
#include <windows.h>
int main()
{
// 打开共享内存区
HANDLE hMapFile = OpenFileMapping(FILE_MAP_ALL_ACCESS, FALSE, "PythonServer");
if (hMapFile == NULL)
{
std::cerr << "Failed to open shared memory area!" << std::endl;
return 1;
}
// 映射共享内存到本地内存
LPVOID lpBase = MapViewOfFile(hMapFile, FILE_MAP_ALL_ACCESS, 0, 0, 1024);
if (lpBase == NULL)
{
std::cerr << "Failed to map shared memory area!" << std::endl;
CloseHandle(hMapFile);
return 1;
}
// 向服务器发送数据
const char* data = "World";
memcpy(lpBase, data, strlen(data) + 1);
// 等待服务器返回数据
Sleep(1000);
char response[1024];
memcpy(response, lpBase, 1024);
std::cout << "Received response: " << response << std::endl;
// 关闭共享内存区
UnmapViewOfFile(lpBase);
CloseHandle(hMapFile);
return 0;
}
```
注意:在Windows下使用共享内存通信需要使用Windows API相关函数,因此上述代码只能在Windows环境下运行。另外,共享内存的大小需要事先确定,并且在服务器和客户端之间需要协商好数据的格式和传输方式。
阅读全文
相关推荐
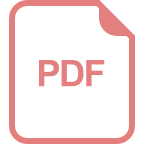
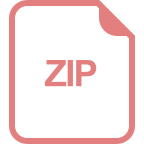
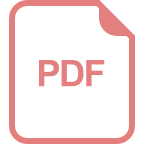
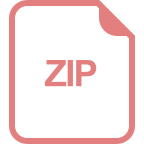
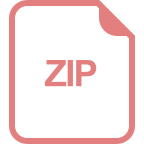
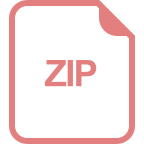
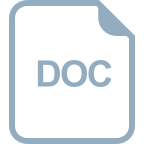
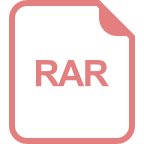
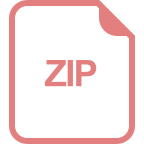
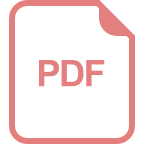
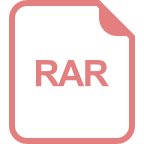
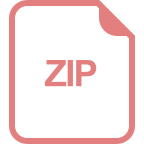
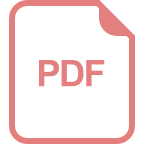
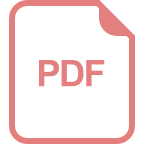
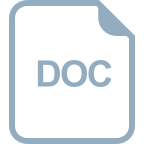
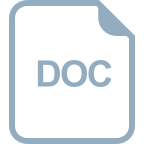
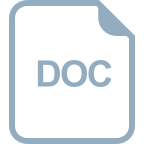