输入一行字符,以#为结尾,输出其中数字的和,
时间: 2024-04-29 10:19:28 浏览: 28
好的,可以为您提供一段 Python 代码来实现这个功能:
```python
def sum_digits_until_hashtag():
s = input("请输入一行字符,以#为结尾:")
total = 0
while s[-1] != "#":
s += input()
for c in s[:-1]:
if c.isdigit():
total += int(c)
return total
```
这个函数会要求用户输入一行字符,直到以#结尾。然后它会计算这行字符中所有数字的总和,并返回结果。例如:
```python
>>> sum_digits_until_hashtag()
请输入一行字符,以#为结尾:hello 123 world 456#
21
```
在这个示例中,用户输入的字符是 "hello 123 world 456#",其中的数字是 1、2、3、4、5 和 6,它们的总和是 21。
相关问题
输入一行字符,以#结尾,分别统计出其中的英文字母、空格、数字和其他字符的个数(不算#号)。
回车键结束,统计其中英文字母、空格、数字和其他字符的个数。
首先,需要定义四个变量,分别用于存储英文字母、空格、数字和其他字符的个数。然后,使用循环读取输入的字符,判断每个字符属于哪一类,并将对应的变量加1。最后,输出四个变量的值即可。
示例代码:
```
#include <stdio.h>
int main() {
int letter = 0, space = 0, digit = 0, other = 0;
char ch;
printf("请输入一行字符:\n");
while ((ch = getchar()) != '\n') {
if (ch >= 'a' && ch <= 'z' || ch >= 'A' && ch <= 'Z') {
letter++;
} else if (ch == ' ') {
space++;
} else if (ch >= '0' && ch <= '9') {
digit++;
} else {
other++;
}
}
printf("英文字母个数:%d\n", letter);
printf("空格个数:%d\n", space);
printf("数字个数:%d\n", digit);
printf("其他字符个数:%d\n", other);
return 0;
}
```
C语言输入一行字符,把其中的非数字存到数组中输出
可以使用C语言的标准库函数`isdigit()`来判断一个字符是否为数字。具体实现过程如下:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char input[100]; // 定义输入数组
char non_digits[100]; // 定义非数字数组
int i = 0, j = 0;
printf("请输入一行字符:\n");
fgets(input, 100, stdin); // 从标准输入中读取一行字符
while (input[i] != '\0') { // 遍历输入数组
if (!isdigit(input[i])) { // 如果当前字符不是数字
non_digits[j] = input[i]; // 将非数字存入非数字数组
j++;
}
i++;
}
printf("非数字字符为:\n");
printf("%s", non_digits); // 输出非数字数组
return 0;
}
```
需要注意的是,在输入字符时要使用`fgets()`函数,而不是`scanf()`函数,因为`fgets()`可以读取一整行字符,而不会因为空格或换行符而中断读取。同时,读取的字符数组最后会自动加上一个`\0`作为结尾标志,因此我们可以通过判断是否到达了结尾来结束遍历。
相关推荐
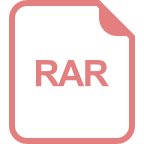












