C语言amr转成mp3格式
时间: 2023-07-09 12:13:08 浏览: 79
要在C语言中将AMR文件转换为MP3格式,你需要使用第三方库来完成。以下是一些常用的库和方法:
1. 使用FFmpeg库:FFmpeg是一个免费的开源软件,可以用于处理音频和视频文件。你可以使用libavcodec库来将AMR文件转换为MP3格式。首先需要安装FFmpeg库,然后使用以下代码来进行转换:
```
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/avutil.h>
int main()
{
AVFormatContext *fmt_ctx = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVPacket pkt;
AVFrame *frame = NULL;
int ret, i;
av_register_all();
avcodec_register_all();
// open input file
if ((ret = avformat_open_input(&fmt_ctx, "input.amr", NULL, NULL)) < 0) {
fprintf(stderr, "Cannot open input file\n");
return ret;
}
// find audio stream info
if ((ret = avformat_find_stream_info(fmt_ctx, NULL)) < 0) {
fprintf(stderr, "Cannot find stream information\n");
return ret;
}
// find audio decoder
codec = avcodec_find_decoder(fmt_ctx->streams[0]->codecpar->codec_id);
if (!codec) {
fprintf(stderr, "Unsupported codec\n");
return -1;
}
// allocate codec context
codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
fprintf(stderr, "Cannot allocate codec context\n");
return -1;
}
// copy codec parameters
if ((ret = avcodec_parameters_to_context(codec_ctx, fmt_ctx->streams[0]->codecpar)) < 0) {
fprintf(stderr, "Cannot copy codec parameters\n");
return ret;
}
// open codec
if ((ret = avcodec_open2(codec_ctx, codec, NULL)) < 0) {
fprintf(stderr, "Cannot open codec\n");
return ret;
}
// allocate frame
frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Cannot allocate frame\n");
return -1;
}
// initialize packet
av_init_packet(&pkt);
pkt.data = NULL;
pkt.size = 0;
// open output file
FILE *outfile = fopen("output.mp3", "wb");
if (!outfile) {
fprintf(stderr, "Cannot open output file\n");
return -1;
}
// read frames from input file
while (av_read_frame(fmt_ctx, &pkt) >= 0) {
// decode frame
ret = avcodec_send_packet(codec_ctx, &pkt);
if (ret < 0) {
fprintf(stderr, "Error sending packet for decoding\n");
return ret;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
fprintf(stderr, "Error during decoding\n");
return ret;
}
// encode frame
AVCodecContext *enc_ctx;
AVCodec *enc;
AVPacket enc_pkt;
av_init_packet(&enc_pkt);
enc_pkt.data = NULL;
enc_pkt.size = 0;
enc = avcodec_find_encoder(AV_CODEC_ID_MP3);
if (!enc) {
fprintf(stderr, "Codec not found\n");
return -1;
}
enc_ctx = avcodec_alloc_context3(enc);
if (!enc_ctx) {
fprintf(stderr, "Cannot allocate encoder context\n");
return -1;
}
enc_ctx->bit_rate = codec_ctx->bit_rate;
enc_ctx->sample_rate = codec_ctx->sample_rate;
enc_ctx->channels = codec_ctx->channels;
enc_ctx->channel_layout = codec_ctx->channel_layout;
enc_ctx->sample_fmt = enc->sample_fmts[0];
enc_ctx->flags |= AV_CODEC_FLAG_GLOBAL_HEADER;
if ((ret = avcodec_open2(enc_ctx, enc, NULL)) < 0) {
fprintf(stderr, "Cannot open encoder\n");
return ret;
}
ret = avcodec_send_frame(enc_ctx, frame);
if (ret < 0) {
fprintf(stderr, "Error sending frame for encoding\n");
return ret;
}
while (ret >= 0) {
ret = avcodec_receive_packet(enc_ctx, &enc_pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
fprintf(stderr, "Error during encoding\n");
return ret;
}
// write packet to output file
fwrite(enc_pkt.data, 1, enc_pkt.size, outfile);
av_packet_unref(&enc_pkt);
}
avcodec_free_context(&enc_ctx);
}
av_packet_unref(&pkt);
}
// close input file
avformat_close_input(&fmt_ctx);
// close output file
fclose(outfile);
// free resources
avcodec_free_context(&codec_ctx);
av_frame_free(&frame);
return 0;
}
```
2. 使用其他第三方库:除了FFmpeg库,还有其他一些库可以用于音频转换,如lame、libmp3lame等。你可以根据自己的需求选择适合自己的库。
需要注意的是,使用第三方库需要了解其使用方法和相关知识,建议在使用前仔细阅读文档和示例代码。
相关推荐
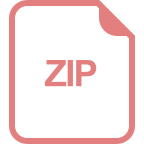















