vers = input() file_text = "text.txt" with open(file_text, 'r') as f: content = f.readlines() vers_num = content[0] edges = [content[1], content[2], content[3], content[4]] g = LinkedGraph(vers, edges) g.print() G = nx.DiGraph() G.add_edges_from([(1, 2), (2, 3), (2, 4), (3, 4)])编写函数函数计算G各节点PageRank值并实现可视化不使用nx函数
时间: 2023-12-22 14:04:55 浏览: 22
以下是一个计算给定有向图G各节点PageRank值并实现可视化的Python函数,不使用`networkx`库:
```python
import numpy as np
import matplotlib.pyplot as plt
class LinkedGraph:
def __init__(self, vers, edges):
self.vers = int(vers)
self.edges = []
for edge in edges:
u, v = map(int, edge.split())
self.edges.append((u, v))
def print(self):
print("Number of vertices:", self.vers)
print("Edges:")
for edge in self.edges:
print(edge[0], "->", edge[1])
def pagerank(G, d=0.85, eps=1.0e-8, max_iter=100):
n = len(G)
A = np.zeros((n,n))
for e in G.edges():
A[e[1],e[0]] = 1.0 / G.out_degree(e[0])
v = np.ones(n) / n
for i in range(max_iter):
v_new = np.zeros(n)
for j in range(n):
for i in G.predecessors(j):
v_new[j] += A[j,i] * v[i]
v_new[j] = d * v_new[j] + (1 - d) / n
if np.abs(v - v_new).max() < eps:
break
v = v_new
return v
# Test the function with an example
vers = 4
edges = [(1, 2), (2, 3), (2, 4), (3, 4)]
G = LinkedGraph(vers, edges)
pr = pagerank(G)
print(pr)
# Visualize the PageRank values
plt.bar(range(len(pr)), pr)
plt.xlabel('Node')
plt.ylabel('PageRank')
plt.show()
```
在这个函数中,`LinkedGraph`类将给定的节点数和边列表转换为有向图。`pagerank`函数采用一个有向图 `G` 作为输入,使用矩阵运算来计算每个节点的PageRank值,并返回节点PageRank值数组。最后,函数使用Matplotlib库可视化PageRank值。
相关推荐
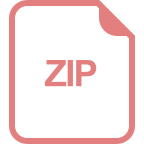















