使用c++的引用,注意提交时把代码选为c++;在主函数定义字符指针 char *p,然后在子函数内malloc申请空间,通过fgets读取字符串,然后在主函数中进行输出;要求子函数使用c++的引用,注意在c++中从标准输入读取字符串,需要使用fgets(p,100,stdin)
时间: 2023-05-01 20:02:07 浏览: 137
这段代码使用了C++语言,建议在主函数中定义字符指针 char *p,并在子函数中使用 malloc 申请空间,在使用 fgets 读取字符串,并在主函数中输出。需要注意的是,使用 C++ 的引用,注意在 C++ 中从标准输入输出读取字符串需要使用 fgets(p,100,stdin) 函数。
相关问题
如果函数形参类型为char *,该怎么通过动态内存分配使可以直接传入const char *的值?使用C++语言,来给出一个具体的例子
可以使用C++中的类型转换和动态内存分配来实现将const char *传入char *类型的函数参数。具体实现步骤如下:
1. 在函数中将char *类型的形参声明为const char *类型,以便接受const char *类型的实参。
2. 使用动态内存分配函数如malloc、new等申请一块内存空间,大小为传入的字符串长度加1(以存放字符串结束符'\0')。
3. 将传入的字符串复制到动态分配的内存空间中。
4. 返回动态分配的内存空间的指针。
下面是一个具体的例子:
```c++
#include <iostream>
#include <cstring>
using namespace std;
// 函数形参类型为const char *
char *test(const char *str)
{
// 动态分配内存空间
char *p = new char[strlen(str) + 1];
// 复制传入的字符串到动态分配的内存空间
strcpy(p, str);
// 返回动态分配的内存空间的指针
return p;
}
int main()
{
const char *str = "Hello World!";
char *p = test(str);
cout << p << endl;
// 释放动态分配的内存空间
delete [] p;
return 0;
}
```
在这个例子中,我们定义了一个函数test,它的形参类型为const char *。在函数内部,我们使用new关键字申请了一块内存空间,大小为传入字符串的长度加1。然后,我们使用strcpy函数将传入的字符串复制到动态分配的内存空间中,并返回指向这块内存空间的指针。在主函数中,我们定义了一个const char *类型的字符串,然后调用test函数,并将返回的指针赋值给char *类型的指针p。最后,我们输出p指向的字符串,并使用delete关键字释放动态分配的内存空间。
使用c或者c++,封装一个类型转换的函数,把所有类型转换为char*,函数的形参是可变的,注释为简体中文
以下是一个简单的封装类型转换为 char* 的函数,可以接受可变数量和类型的参数:
```c++
#include <stdarg.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char* convert_to_char(const char* format, ...)
{
va_list args;
va_start(args, format);
// 计算输出字符串长度
int length = vsnprintf(NULL, 0, format, args) + 1;
// 分配字符串内存
char* result = (char*)malloc(length);
// 重新开始变量参数列表
va_start(args, format);
// 将输入值转换为字符串
vsnprintf(result, length, format, args);
// 关闭变量参数列表
va_end(args);
return result;
}
```
该函数使用了可变参数列表,将输入值转换为字符串,并返回一个指向转换后的字符串的指针。
示例用法:
```c++
int main()
{
int num = 10;
float fnum = 3.14;
char* str = "Hello World!";
char* result = convert_to_char("数字:%d 浮点数:%f 字符串:%s", num, fnum, str);
printf("%s\n", result);
free(result);
return 0;
}
```
输出结果为:
```
数字:10 浮点数:3.140000 字符串:Hello World!
```
阅读全文
相关推荐
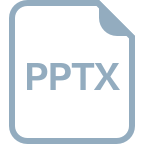
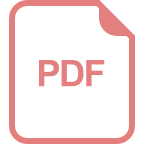
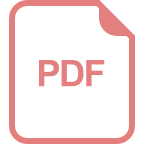









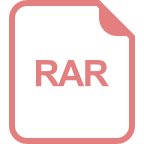
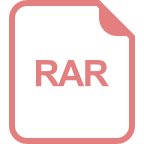
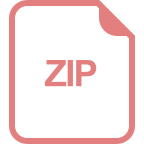
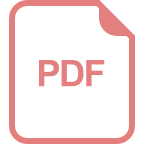
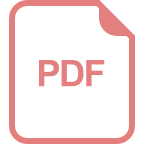