写一个程序从输入文件中去读取四行大写字母(全都是大写的,每行不超过 100 100 个字符),然后用柱状图输出每个字符在输入文件中出现的次数。 输入格式 四行字符,由大写字母组成,每行不超过 100 100 个字符
时间: 2024-09-29 08:04:19 浏览: 22
为了实现这个程序,你可以使用Python的内置库`collections`中的`Counter`类以及`matplotlib`库来绘制柱状图。这里是一个简单的步骤说明:
首先,安装必要的库(如果尚未安装):
```bash
pip install collections matplotlib
```
接下来,编写Python代码:
```python
import collections
import matplotlib.pyplot as plt
# 读取输入文件
def read_input_file(file_path):
with open(file_path, 'r') as file:
lines = file.read().splitlines()[:4] # 只取前四行
return [line.strip() for line in lines if line.isupper()] # 去除空格并只保留大写字母
# 统计字符出现次数
def count_chars(lines):
char_counts = collections.Counter(''.join(lines))
return char_counts
# 绘制柱状图
def plot_char_histogram(char_counts):
labels, values = zip(*char_counts.items()) # 分离标签和值
plt.bar(labels, values)
plt.xlabel('Characters')
plt.ylabel('Frequency')
plt.title('Character Frequency in Input File')
plt.show()
# 主函数
def main():
file_path = "input.txt" # 替换为你实际的文件路径
input_lines = read_input_file(file_path)
char_counts = count_chars(input_lines)
plot_char_histogram(char_counts)
if __name__ == "__main__":
main()
```
在这个程序中,`read_input_file` 函数负责读取文件并处理数据,`count_chars` 函数统计字符频率,而 `plot_char_histogram` 则用于可视化结果。记得替换 "input.txt" 为你的输入文件路径。
阅读全文
相关推荐
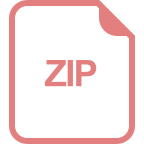
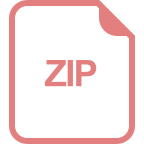
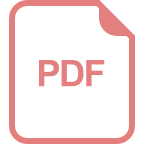















