请使用stm32f407的库函数为我写一份矩阵键盘的代码
时间: 2023-06-07 17:07:28 浏览: 94
好的,我会根据您的要求为您提供矩阵键盘的代码。以下是代码示例:
```
#include "stm32f4xx.h"
//定义矩阵键盘端口和引脚
#define ROW_GPIO GPIOA
#define ROW_PIN GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3
#define COL_GPIO GPIOB
#define COL_PIN GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3
//矩阵键盘按键对应的键值
const uint8_t key_map[4][4] = {
{1, 2, 3, 10},
{4, 5, 6, 11},
{7, 8, 9, 12},
{15, 0, 14, 13}
};
void init_matrix_keypad(void) {
//初始化矩阵键盘端口
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA | RCC_AHB1Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Pin = ROW_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(ROW_GPIO, &GPIO_InitStructure);
GPIO_SetBits(ROW_GPIO, ROW_PIN);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_Pin = COL_PIN;
GPIO_Init(COL_GPIO, &GPIO_InitStructure);
GPIO_SetBits(COL_GPIO, COL_PIN);
}
uint8_t read_matrix_keypad(void) {
uint8_t i, j, result;
//扫描矩阵键盘
for (i = 0; i < 4; i++) {
GPIO_ResetBits(ROW_GPIO, 1 << i);
for (j = 0; j < 4; j++) {
if (GPIO_ReadInputDataBit(COL_GPIO, 1 << j) == RESET) {
while (GPIO_ReadInputDataBit(COL_GPIO, 1 << j) == RESET) {}
result = key_map[i][j];
GPIO_SetBits(ROW_GPIO, 1 << i);
return result;
}
}
GPIO_SetBits(ROW_GPIO, 1 << i);
}
return 0xFF;
}
int main(void) {
init_matrix_keypad();
while (1) {
uint8_t key = read_matrix_keypad();
if (key != 0xFF) {
//获取到按键值,执行对应操作
//do something
}
}
}
```
以上是一个简单的基于STM32F407的矩阵键盘代码示例,您可以根据自己的需求进行修改和优化。
阅读全文
相关推荐
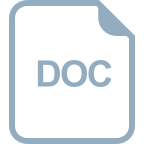
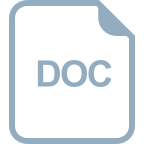
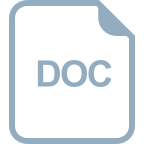
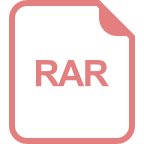
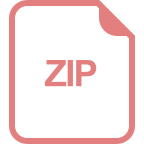
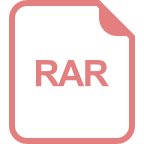
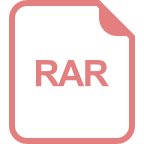
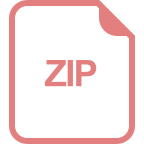
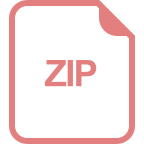
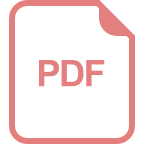
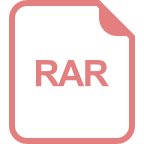
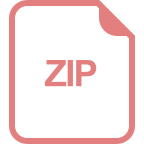
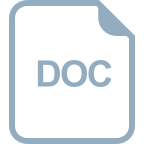
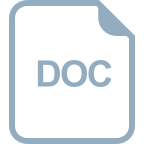
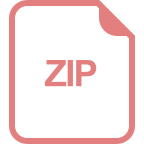
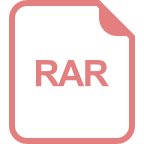
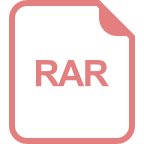