Mat faceROI = frame_gray( faces[i] );
时间: 2024-05-28 09:15:26 浏览: 132
This line of code creates a new variable named "faceROI" of type "Mat" (short for "matrix"), which will store a portion of the grayscale input image "frame_gray". The portion of the image to be stored is defined by the rectangular region specified by "faces[i]", which is a vector of detected faces (likely obtained using a face detection algorithm).
In other words, "faceROI" will store the grayscale pixels corresponding to the i-th detected face in the input image. This region of the image can then be processed separately from the rest of the image, for example, to apply facial recognition or other image analysis techniques.
相关问题
void detectAndDisplay(Mat frame) { Mat frame_gray; cvtColor(frame, frame_gray, COLOR_BGR2GRAY); equalizeHist(frame_gray, frame_gray); //-- Detect faces std::vector<Rect> faces; face_cascade.detectMultiScale(frame_gray, faces); for (size_t i = 0; i < faces.size(); i++) { Point center(faces[i].x + faces[i].width / 2, faces[i].y + faces[i].height / 2); ellipse(frame, center, Size(faces[i].width / 2, faces[i].height / 2), 0, 0, 360, Scalar(255, 0, 255), 4); Mat faceROI = frame_gray(faces[i]); //-- In each face, detect eyes std::vector<Rect> eyes; eyes_cascade.detectMultiScale(faceROI, eyes); for (size_t j = 0; j < eyes.size(); j++) { Point eye_center(faces[i].x + eyes[j].x + eyes[j].width / 2, faces[i].y + eyes[j].y + eyes[j].height / 2); int radius = cvRound((eyes[j].width + eyes[j].height) * 0.25); circle(frame, eye_center, radius, Scalar(255, 0, 0), 4); } } //-- Show what you got imshow("Capture - Face detection", frame); }
这段代码是一个函数,用于检测输入图像中的人脸和眼睛,并在图像上进行标记。该函数的输入参数是一个Mat类型的图像帧,函数会将其转换为灰度图像,并进行直方图均衡化以增强图像对比度。接着,使用人脸检测分类器face_cascade对图像中的人脸进行检测,得到人脸的位置和大小信息。对于每个检测到的人脸,函数会在图像上绘制椭圆形标记,并使用眼部检测分类器eyes_cascade对人脸区域进行眼睛检测,得到眼睛的位置和大小信息。对于每个检测到的眼睛,函数会在图像上绘制圆形标记。最后,函数会在图像窗口中显示标记后的图像。
该函数的主要功能是人脸和眼睛检测,并将检测结果在图像中进行标记。可以在图像处理和计算机视觉领域的应用中使用,如人脸识别、安防监控等。
改正代码#include <opencv2/opencv.hpp> #include <opencv2/face.hpp> #include <iostream> using namespace std; using namespace cv; using namespace cv::face; int main() { // 加载人脸检测器和人脸识别器 CascadeClassifier faceCascade; faceCascade.load("haarcascade_frontalface_default.xml"); Ptr<LBPHFaceRecognizer> recognizer = LBPHFaceRecognizer::create(); recognizer->read("face_recognizer.xml"); // 打开摄像头 VideoCapture cap(0); if (!cap.isOpened()) { cout << "无法打开摄像头" << endl; return -1; } // 循环捕获图像并进行处理 while (true) { Mat frame; cap >> frame; // 将图像转换为灰度图像并进行直方图均衡化 Mat gray; cvtColor(frame, gray, COLOR_BGR2GRAY); equalizeHist(gray, gray); // 使用人脸检测器检测人脸 vector<Rect> faces; faceCascade.detectMultiScale(gray, faces, 1.1, 2, 0 | CASCADE_SCALE_IMAGE, Size(30, 30)); // 在图像中标记人脸 for (size_t i = 0; i < faces.size(); i++) { Rect face = faces[i]; rectangle(frame, face, Scalar(0, 0, 255), 2); // 对每个人脸进行识别 Mat faceROI = gray(face); int label = -1; double confidence = 0; recognizer->predict(faceROI, label, confidence); // 显示识别结果 string text = format("Person %d (%.2f)", label, confidence); putText(frame, text, Point(face.x, face.y - 10), FONT_HERSHEY_SIMPLEX, 0.7, Scalar(0, 0, 255), 2); } // 显示图像并等待按键 imshow("Face Recognition", frame); if (waitKey(30) == 27) break; } return 0; }
代码中没有问题,但是需要注意以下几点:
1. 需要确保已经下载好了人脸检测器和人脸识别器的模型文件,分别为 haarcascade_frontalface_default.xml 和 face_recognizer.xml。
2. 在使用人脸检测器进行人脸检测时,需要设置合适的 scaleFactor 和 minNeighbors 参数,以便对不同大小和姿态的人脸都能进行检测。
3. 在使用 LBPHFaceRecognizer 进行人脸识别时,需要先使用 train 函数对模型进行训练,并将训练好的模型保存到文件中,以便后续进行预测。
阅读全文
相关推荐
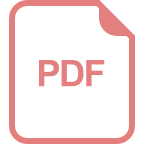
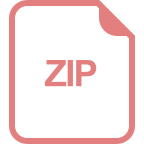
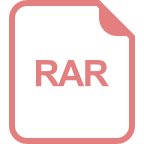







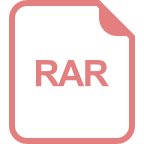
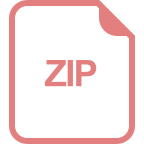
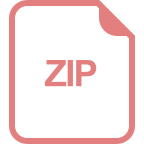