org.openxmlformats.schemas.wordprocessingml.x2006.main.CTTc 获取列字符串字体类型,是否加粗,字体大小
时间: 2023-11-12 14:06:10 浏览: 218
要列的字符串字体类型、是否加粗和字体大小,您可以使用Apache POI库中的`XWPFTableCell`类和OpenXML的CTTc(列定义)对象。以下是一个示例代码,演示如何获取列的字体类型、是否加粗和字体大小:
```java
import org.apache.poi.xwpf.usermodel.*;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.*;
import java.io.FileInputStream;
import java.io.IOException;
public class WordTableFormatReader {
public static void main(String[] args) {
try {
// 读取Word文档
FileInputStream fileInputStream = new FileInputStream("input.docx");
XWPFDocument document = new XWPFDocument(fileInputStream);
fileInputStream.close();
// 获取第一个表格
XWPFTable table = document.getTables().get(0); // 假设文档中只有一个表格
// 获取表格列数
int columnCount = table.getRow(0).getTableCells().size();
// 遍历表格列的格式
for (int columnIndex = 0; columnIndex < columnCount; columnIndex++) {
// 获取第一行的单元格
XWPFTableCell cell = table.getRow(0).getCell(columnIndex);
// 获取CTTc对象
CTTc ctTc = cell.getCTTc();
// 获取列的字体类型
if (ctTc.isSetTcPr()) {
CTTcPr tcPr = ctTc.getTcPr();
if (tcPr.isSetPPr()) {
CTPPr pPr = tcPr.getPPr();
if (pPr.isSetRPr()) {
CTRPr rPr = pPr.getRPr();
if (rPr.isSetRFonts()) {
CTFonts fonts = rPr.getRFonts();
String fontFamily = fonts.getAscii();
System.out.println("列 " + (columnIndex + 1) + " 的字体类型: " + fontFamily);
}
}
}
}
// 获取列的是否加粗属性
boolean isBold = false;
if (ctTc.isSetTcPr()) {
CTTcPr tcPr = ctTc.getTcPr();
if (tcPr.isSetPPr()) {
CTPPr pPr = tcPr.getPPr();
if (pPr.isSetRPr()) {
CTRPr rPr = pPr.getRPr();
isBold = rPr.isSetB() && rPr.getB().isVal();
}
}
}
System.out.println("列 " + (columnIndex + 1) + " 是否加粗: " + isBold);
// 获取列的字体大小
int fontSize = -1;
if (ctTc.isSetTcPr()) {
CTTcPr tcPr = ctTc.getTcPr();
if (tcPr.isSetPPr()) {
CTPPr pPr = tcPr.getPPr();
if (pPr.isSetRPr()) {
CTRPr rPr = pPr.getRPr();
if (rPr.isSetSz()) {
CTHpsMeasure sz = rPr.getSz();
fontSize = sz.getVal().intValue();
}
}
}
}
System.out.println("列 " + (columnIndex + 1) + " 的字体大小: " + fontSize);
}
System.out.println("表格列格式读取成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们首先使用`FileInputStream`来读取名为"input.docx"的Word文档,然后使用`XWPFDocument`类加载文档内容。
接下来,我们使用`document.getTables().get(0)`获取文档中的第一个表格。如果您的文档中有多个表格,请根据实际情况选择要读取格式的表格。
然后,我们使用`table.getRow(0).getTableCells().size()`获取表格的列数。这里假设第一行中的单元格数与其他行相同。
接下来,我们使用一个循环来遍历每一列,并获取每个单元格的CTTc对象。然后,我们检查CTTc对象是否设置了TcPr(列属性)。如果设置了TcPr,我们进一步获取PPr(段落属性),RPr(运行属性)和RFonts(字体属性)等对象,并从中获取字体类型。
我们还检查是否设置了B(加粗)属性和Sz(字体大小)属性,并从中获取相应的值。
请确保在代码中引入了正确的POI库以及其他所需的依赖项,并且在代码中导入了正确的类和包。
阅读全文
相关推荐
















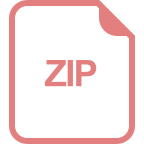