vue3封装一个el-table组件,功能齐全
时间: 2023-11-17 08:14:06 浏览: 170
下面是一个基于 Vue3 的 el-table 组件的封装示例,包含了常用的功能:
```html
<template>
<el-table
:data="tableData"
:height="height"
:stripe="stripe"
:border="border"
:fit="fit"
:show-header="showHeader"
:highlight-current-row="highlightCurrentRow"
:row-class-name="rowClassName"
:row-style="rowStyle"
:empty-text="emptyText"
:loading="loading"
:default-sort="defaultSort"
:row-key="rowKey"
:size="size"
:cell-class-name="cellClassName"
:cell-style="cellStyle"
@select="handleSelect"
@select-all="handleSelectAll"
@sort-change="handleSortChange"
>
<el-table-column
v-for="column in columns"
:key="column.prop"
:prop="column.prop"
:label="column.label"
:width="column.width"
:min-width="column.minWidth"
:resizable="column.resizable"
:show-overflow-tooltip="column.showOverflowTooltip"
:fixed="column.fixed"
:formatter="column.formatter"
:align="column.align"
:header-align="column.headerAlign"
:sortable="column.sortable"
:sort-method="column.sortMethod"
:sort-by="column.sortBy"
:sort-orders="column.sortOrders"
:render-header="column.renderHeader"
>
<!-- 自定义列内容 -->
<template v-if="column.slot" v-slot:[`header.${column.prop}`]>
{{ column.label }}
</template>
<template v-if="column.slot" v-slot:[`default.${column.prop}`]="{ row }">
<slot :name="column.slot" :row="row" />
</template>
</el-table-column>
</el-table>
</template>
<script>
import { ref } from 'vue';
export default {
name: 'MyTable',
props: {
columns: {
type: Array,
required: true,
},
data: {
type: Array,
required: true,
},
height: {
type: [Number, String],
default: 'auto',
},
stripe: {
type: Boolean,
default: false,
},
border: {
type: Boolean,
default: false,
},
fit: {
type: Boolean,
default: true,
},
showHeader: {
type: Boolean,
default: true,
},
highlightCurrentRow: {
type: Boolean,
default: false,
},
rowClassName: {
type: Function,
default: () => '',
},
rowStyle: {
type: Function,
default: () => ({}),
},
emptyText: {
type: String,
default: '暂无数据',
},
loading: {
type: Boolean,
default: false,
},
defaultSort: {
type: Object,
default: null,
},
rowKey: {
type: [String, Function],
default: 'id',
},
size: {
type: String,
default: 'medium',
},
cellClassName: {
type: Function,
default: () => '',
},
cellStyle: {
type: Function,
default: () => ({}),
},
},
setup(props, context) {
const tableData = ref(props.data);
// 处理选择事件
const handleSelect = (selection, row) => {
context.emit('select', selection, row);
};
// 处理全选事件
const handleSelectAll = (selection) => {
context.emit('select-all', selection);
};
// 处理排序事件
const handleSortChange = (sort) => {
context.emit('sort-change', sort);
};
return {
tableData,
handleSelect,
handleSelectAll,
handleSortChange,
};
},
};
</script>
```
在使用时,只需要传入 columns 和 data 两个 props 即可:
```html
<template>
<my-table :columns="columns" :data="tableData" />
</template>
<script>
import MyTable from '@/components/MyTable.vue';
export default {
components: {
MyTable,
},
data() {
return {
columns: [
{
prop: 'name',
label: '姓名',
},
{
prop: 'age',
label: '年龄',
},
{
prop: 'gender',
label: '性别',
},
],
tableData: [
{
name: '张三',
age: 18,
gender: '男',
},
{
name: '李四',
age: 20,
gender: '女',
},
{
name: '王五',
age: 22,
gender: '男',
},
],
};
},
};
</script>
```
阅读全文
相关推荐
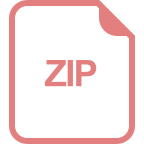
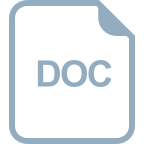







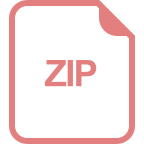
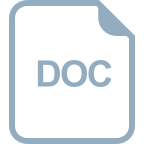
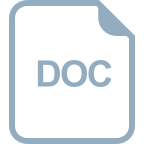
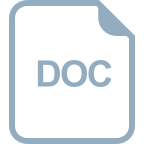



