android ble hid
时间: 2023-05-17 17:00:46 浏览: 396
Android BLE HID指的是Android系统中实现BLE(蓝牙低功耗)的Human Interface Device(人机界面设备)功能。通过BLE HID,Android设备可以与BLE HID设备(例如键盘、鼠标、手柄等)建立低功耗蓝牙连接,并且可以通过蓝牙无线技术实现设备的数据传输和控制。
在Android系统中,BLE HID功能的实现需要使用Android的BLE API,包括BluetoothGatt、BluetoothDevice、BluetoothGattCharacteristic和BluetoothGattDescriptor等类。其中,BluetoothGattCharacteristic类可以表示BLE设备的特征,例如键盘上的按键、鼠标上的光标移动等,而BluetoothGattDescriptor则可以表示特征的属性,例如属性的读写权限、属性的值等等。
通过使用Android的BLE HID功能,可以将BLE HID设备与Android设备进行配对,并通过蓝牙技术进行数据传输和控制,提高设备之间的互动性和便捷性。例如,在游戏中使用蓝牙手柄,可以更加自由地控制角色的移动和攻击,提高游戏体验。
总之,Android BLE HID是一项非常有用的技术,可以让Android设备与BLE HID设备之间进行无线连接,实现数据传输和控制,提高设备之间的互动性和便捷性。
相关问题
Android 实现手机与蓝牙BLE HID设备的配对连接,解绑,断开连接的代码
以下是 Android 实现手机与蓝牙BLE HID设备的配对连接、解绑、断开连接的代码:
1. 配对连接
```java
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress);
BluetoothGatt gatt = device.connectGatt(context, false, gattCallback, BluetoothDevice.TRANSPORT_LE);
// 发现服务
gatt.discoverServices();
// 连接状态回调
private final BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
super.onConnectionStateChange(gatt, status, newState);
if (newState == BluetoothProfile.STATE_CONNECTED) {
// 连接成功,发现服务
gatt.discoverServices();
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 连接断开
gatt.close();
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
super.onServicesDiscovered(gatt, status);
if (status == BluetoothGatt.GATT_SUCCESS) {
// 发现服务成功
BluetoothGattService service = gatt.getService(SERVICE_UUID);
BluetoothGattCharacteristic characteristic = service.getCharacteristic(CHARACTERISTIC_UUID);
// 读取特征值
gatt.readCharacteristic(characteristic);
}
}
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
super.onCharacteristicRead(gatt, characteristic, status);
if (status == BluetoothGatt.GATT_SUCCESS) {
// 读取特征值成功
byte[] value = characteristic.getValue();
// 处理特征值
handleCharacteristicValue(value);
}
}
};
```
2. 解绑
```java
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress);
BluetoothGatt gatt = device.connectGatt(context, false, gattCallback, BluetoothDevice.TRANSPORT_LE);
// 断开连接
gatt.disconnect();
// 关闭连接
gatt.close();
```
3. 断开连接
```java
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress);
BluetoothGatt gatt = device.connectGatt(context, false, gattCallback, BluetoothDevice.TRANSPORT_LE);
// 断开连接
gatt.disconnect();
```
需要注意的是,以上代码中的 `SERVICE_UUID` 和 `CHARACTERISTIC_UUID` 分别为 BLE HID 设备的服务 UUID 和特征值 UUID,具体值需要根据实际设备进行设置。同时,还需要在 AndroidManifest.xml 文件中添加以下权限:
```
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
```
可以像连接普通BLE设备那样连接HID外设吗?
不可以,连接HID设备是需要遵循HID协议的。HID设备与普通BLE设备不同,它们使用不同的蓝牙协议。HID协议定义了一种标准的设备类别,包括键盘、鼠标、手柄等。HID设备与普通BLE设备的连接方式也不同,HID设备需要使用RFCOMM通信协议,而不是GATT协议。
在Android中,连接HID设备需要使用BluetoothHidDeviceProfile类,该类提供了连接和通信HID设备的接口。您需要创建一个BluetoothHidDeviceProfile对象,并实现BluetoothHidDeviceProfile.ServiceListener接口来接收连接和通信事件。
以下是一个简单的示例代码,用于连接HID设备:
```
public class HidDeviceService extends Service implements BluetoothHidDeviceProfile.ServiceListener {
private BluetoothAdapter mBluetoothAdapter;
private BluetoothHidDevice mBluetoothHidDevice;
@Override
public void onCreate() {
super.onCreate();
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
mBluetoothHidDevice = new BluetoothHidDevice(this, this);
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
if (!mBluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivity(enableBtIntent);
} else {
mBluetoothHidDevice.registerApp(
new BluetoothHidDeviceAppSdpSettings(
"HID Device",
"Android",
"",
BluetoothHidDevice.SUBCLASS1_COMBO,
new byte[]{(byte) 0x22, (byte) 0x24, (byte) 0x2a},
new BluetoothHidDeviceAppSdpSettings.Service[]{new BluetoothHidDeviceAppSdpSettings.Service(
BluetoothHidDevice.SUBCLASS1_COMBO,
BluetoothHidDevice.PROTOCOL_BOOT,
BluetoothHidDevice.SERVICE_NAME,
BluetoothHidDevice.SERVICE_DESCRIPTION,
BluetoothHidDevice.SERVICE_PROVIDER)}));
}
return super.onStartCommand(intent, flags, startId);
}
@Override
public void onServiceStateChanged(BluetoothDevice device, int state) {
// 连接状态变化回调
if (state == BluetoothProfile.STATE_CONNECTED) {
// HID设备已连接
} else if (state == BluetoothProfile.STATE_DISCONNECTED) {
// HID设备已断开连接
}
}
@Override
public void onGetReport(BluetoothDevice device, byte type, byte id, int bufferSize) {
// 获取报告请求回调
}
@Override
public void onSetReport(BluetoothDevice device, byte type, byte id, byte[] data) {
// 设置报告请求回调
}
@Override
public void onSetProtocol(BluetoothDevice device, byte protocol) {
// 设置协议请求回调
}
@Override
public void onIntrData(BluetoothDevice device, byte reportId, byte[] data) {
// 中断包数据回调
}
}
```
以上是一个基本的HID设备服务,它使用BluetoothHidDeviceProfile连接和通信HID设备。您需要在AndroidManifest.xml文件中声明该服务,并在必要的时候请求蓝牙权限。
阅读全文
相关推荐
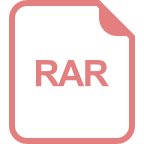
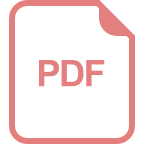
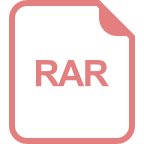
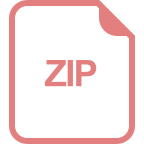
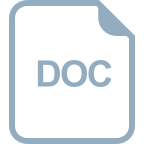
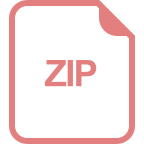
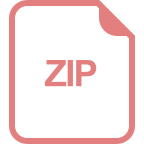
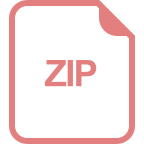
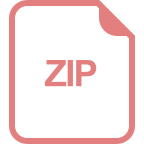
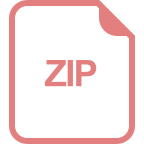
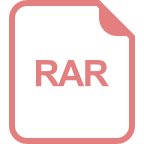
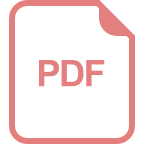
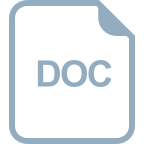
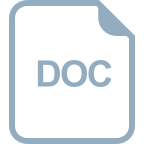