请编写一个c++程序实现统计windows目录下的所有文件大小总和
时间: 2024-05-12 11:17:57 浏览: 8
好的,以下是实现统计Windows目录下所有文件大小总和的C程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
long long int count_size(char *dir_path);
int main(int argc, char **argv)
{
if (argc < 2)
{
printf("Usage: %s <directory path>\n", argv[0]);
return 1;
}
char *dir_path = argv[1];
long long int total_size = count_size(dir_path);
printf("Total size of all files in %s: %lld bytes\n", dir_path, total_size);
return 0;
}
long long int count_size(char *dir_path)
{
DIR *dir = opendir(dir_path);
if (dir == NULL)
{
printf("Error opening directory %s\n", dir_path);
return -1;
}
long long int total_size = 0;
struct dirent *entry;
struct stat info;
char *full_path = malloc(strlen(dir_path) + MAXNAMLEN + 2);
while ((entry = readdir(dir)) != NULL)
{
if (entry->d_name[0] == '.')
continue;
strcpy(full_path, dir_path);
strcat(full_path, "/");
strcat(full_path, entry->d_name);
if (stat(full_path, &info) == -1)
{
printf("Error getting information for file %s\n", full_path);
continue;
}
if (S_ISDIR(info.st_mode))
{
long long int size = count_size(full_path);
total_size += size;
printf("Dir: %s, size: %lld bytes\n", full_path, size);
}
else if (S_ISREG(info.st_mode))
{
total_size += info.st_size;
printf("File: %s, size: %lld bytes\n", full_path, info.st_size);
}
}
free(full_path);
closedir(dir);
return total_size;
}
```
这个程序会递归遍历指定的目录,统计其中所有文件的大小,最终输出总大小。如果目录不存在或打开失败,会输出错误信息并返回-1。如果某个文件的信息获取失败,会输出错误信息并跳过该文件。对于每个文件和目录,程序会输出其路径和大小。
相关推荐
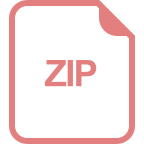
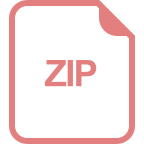














