vue写购物商城的代码
时间: 2024-05-01 17:22:13 浏览: 95
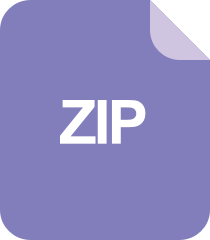
shopping-master.zip
这里提供一个简单的购物商城代码示例,主要使用Vue.js和Bootstrap框架实现:
HTML代码:
```
<div id="app">
<div class="container">
<div class="row">
<div class="col-md-8">
<div class="row">
<div class="col-md-4" v-for="(product, index) in products">
<div class="card mb-4 box-shadow">
<img class="card-img-top" :src="product.image" alt="Card image cap">
<div class="card-body">
<h4 class="card-title">{{product.name}}</h4>
<p class="card-text">{{product.description}}</p>
<div class="d-flex justify-content-between align-items-center">
<span class="price">{{product.price}}</span>
<button class="btn btn-sm btn-outline-secondary" @click="addToCart(index)">Add to cart</button>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="col-md-4">
<div class="card mb-4 box-shadow">
<div class="card-body">
<h4 class="card-title">Cart</h4>
<ul class="list-group" v-if="cart.length">
<li class="list-group-item" v-for="(item, index) in cart">
<span>{{item.name}}</span>
<span class="badge badge-primary badge-pill">{{item.quantity}}</span>
<button class="btn btn-sm btn-danger" @click="removeFromCart(index)">Remove</button>
</li>
</ul>
<div class="alert alert-warning" v-else>
Your cart is empty!
</div>
<p class="total">Total: {{total}}</p>
</div>
</div>
</div>
</div>
</div>
</div>
```
JavaScript代码:
```
var app = new Vue({
el: '#app',
data: {
products: [
{
name: 'Product 1',
description: 'This is a description for Product 1.',
price: 10.99,
image: 'product1.jpg'
},
{
name: 'Product 2',
description: 'This is a description for Product 2.',
price: 19.99,
image: 'product2.jpg'
},
{
name: 'Product 3',
description: 'This is a description for Product 3.',
price: 14.99,
image: 'product3.jpg'
}
],
cart: []
},
methods: {
addToCart: function(index) {
var item = this.products[index];
var cartItem = this.cart.find(function(item) {
return item.name === item.name;
});
if (cartItem) {
cartItem.quantity++;
} else {
this.cart.push({
name: item.name,
price: item.price,
quantity: 1
});
}
},
removeFromCart: function(index) {
this.cart.splice(index, 1);
}
},
computed: {
total: function() {
return this.cart.reduce(function(total, item) {
return total + (item.price * item.quantity);
}, 0);
}
}
});
```
这个示例中,我们使用了Vue.js的数据绑定和计算属性,以及Bootstrap框架的样式。在HTML代码中,我们展示了商品列表和购物车,以及对应的按钮和数量。在JavaScript代码中,我们定义了商品和购物车的数据,并实现了添加和删除商品的方法,以及计算购物车总价的计算属性。
阅读全文
相关推荐
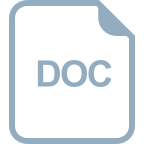
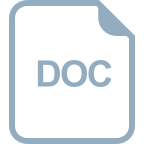
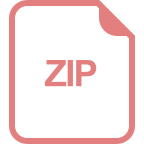
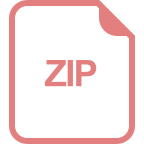
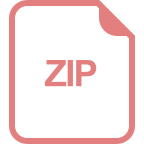
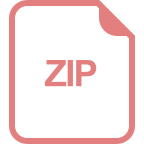
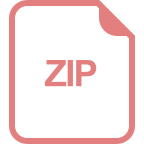
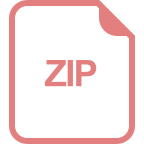
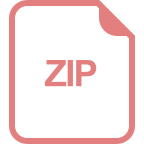
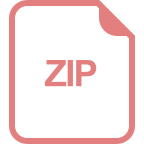
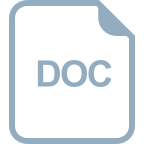
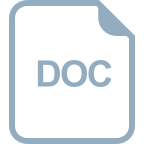
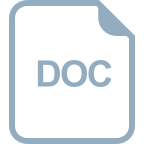




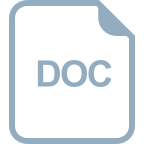
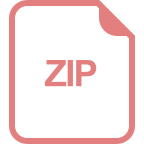