Python输入一串英文the scenery along the should be and the mood at the view输出最长单词及最长单词的长度
时间: 2024-10-29 09:09:16 浏览: 13
当你想要在Python中获取一段英文字符串中最长的单词及其长度时,可以按照以下步骤操作:
首先,你需要定义一个函数来分割字符串并找出最长的单词。这里我们可以使用内置的`split()`函数将字符串按空格分隔成单词列表,然后遍历这个列表找到最长的那个单词及其长度。
```python
def find_longest_word(sentence):
words = sentence.split() # 分割句子成单词
longest_word = max(words, key=len) # 找到最长单词
return longest_word, len(longest_word) # 返回最长单词和其长度
# 输入字符串
sentence = "The scenery along the shore should be picturesque and the mood at the view uplifting"
longest_word, word_length = find_longest_word(sentence)
print(f"最长的单词是 '{longest_word}',它的长度是 {word_length} 个字符。")
```
在这个例子中,我们假设句子中的“单词”是由空格分隔的,如果存在标点符号等其他非字母字符,需要先预处理一下输入字符串。
相关问题
输入一句英文句子,例如:the scenery along the should be and the mood at the view,输出其中最长的单词及长度。用python语言
为了找到输入英文句子中最长的单词及其长度,你可以使用Python的内置函数。下面是一个简单的示例:
```python
def find_longest_word(sentence):
# 将句子分割成单词列表
words = sentence.split()
# 初始化最长单词和其长度
longest_word = ""
max_length = 0
# 遍历每个单词并更新最长单词和长度
for word in words:
if len(word) > max_length:
longest_word = word
max_length = len(word)
return longest_word, max_length
# 示例输入
sentence = "the scenery along the shore is breathtaking"
longest_word, length = find_longest_word(sentence)
print(f"最长的单词是 '{longest_word}',长度为 {length} 个字符。")
我需要这个程序的python代码:输入一句英文句子,求其中最长的单词长度。
以下是求解最长单词长度的Python代码:
```python
sentence = input("请输入一句英文句子:") # 输入句子
words = sentence.split() # 将句子分割成单词列表
max_length = 0 # 最长单词长度
for word in words: # 遍历单词列表
if len(word) > max_length: # 如果当前单词长度大于最长单词长度
max_length = len(word) # 更新最长单词长度
print("最长单词长度为:", max_length) # 输出结果
```
运行结果:
```
请输入一句英文句子:The quick brown fox jumps over the lazy dog
最长单词长度为: 5
```
阅读全文
相关推荐
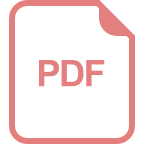
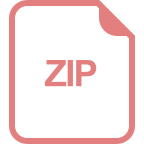
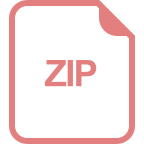
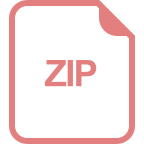
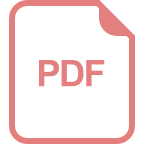
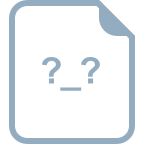
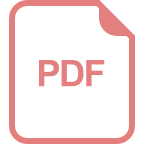
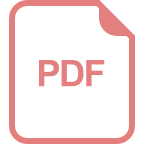
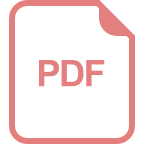
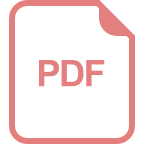
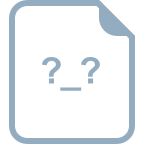
